Introduction
Welcome to an in-depth exploration of ship classification using Convolutional Neural Networks (CNNs) with the Analytics Vidhya hackathon dataset. CNNs are a cornerstone of image-related duties, recognized for his or her means to review hierarchical representations of images. On this endeavor, we dive into understanding the flexibility of CNNs to classify ships primarily based totally on their seen choices.
This endeavor objectives to exhibit deep finding out software program in image categorization and study CNNs constructed from scratch and folks enhanced by the use of change finding out. It explores ship classification, from preprocessing to evaluation and comparability.
Learning Goals
- Apply Convolutional Neural Networks (CNNs) for ship classification.
- Preprocess image information using OpenCV and NumPy.
- Implement CNN fashions every with and with out change finding out.
- Think about model effectivity using metrics like accuracy and F1-score.
- Consider the outcomes of CNN fashions with and with out transfer learning.
Disadvantage Assertion
On this context, the Governmental Maritime and Coastguard Firm seeks to deploy an automated ship detection system leveraging laptop computer imaginative and prescient experience. The goal is to find out and classify ships from images captured by survey boats exactly. With a numerous range of ship types, along with cargo ships, tankers, navy vessels, carriers, and cruise ships, the issue lies in rising a powerful model in a position to distinguishing between these classes efficiently.
Dataset Description
The 5 ship types—Cargo, Tanker, Navy, Service, and Cruise—are represented throughout the dataset by a gaggle of images taken by survey boats. The dataset presents every kind of seen information for model improvement, with 6252 images for teaching and 2680 images for testing.
Clarification of OpenCV and CNN
Permit us to find about OpenCV and CNN intimately:
OpenCV
With its many choices for image processing duties along with object detection, perform extraction, and film enhancing, OpenCV is a potent instrument. We’re in a position to improve top quality of enter images, decide pertinent choices, and preprocess the raw image information by using OpenCV.
Convolutional Neural Networks
CNNs are significantly designed for image-related duties. CNNs are considerably good at robotically extracting choices at different ranges of abstraction from hierarchical representations of images. We’re in a position to create a model which will acknowledge distinctive patterns and traits linked to each sort of ship by teaching a CNN with the labeled ship images.
Layers in CNNs
CNNs embody a lot of layers, each serving a selected aim in processing and extracting choices from enter images. Let’s break down the weather of a CNN:
Convolutional Layers
CNNs are largely composed of convolutional layers. These layers are made up of learnable filters, generally called kernels, which conduct convolution operations on the enter image by sliding over it. The filters use element-wise multiplication and summing operations to extract fully completely different choices from the enter image, along with edges, textures, and patterns. Often, each convolutional layer makes use of a wide range of filters to assemble different choices.
Activation Function
In order so as to add non-linearity to the neighborhood, an activation carry out is utilized element-by-element to the output perform maps following the convolution operation. Tanh, sigmoid, and ReLU (Rectified Linear Unit) are examples of widespread activation options. ReLU’s ease of use and effectivity in fixing the vanishing gradient drawback make it basically probably the most also used activation carry out in CNNs.
Pooling Layers
Pooling layers downsample the perform maps produced by the convolutional layers to guard important information whereas reducing their spatial dimensions. Probably the most well-liked pooling course of, max pooling, efficiently highlights salient choices by retaining the utmost price inside each pooling space. Pooling the enter reduces the neighborhood’s computational complexity and enhances its means to review robust traits, making it further resilient to slight spatial fluctuations.
Completely Linked Layers
Completely linked layers normally perform classification or regression duties primarily based totally on the realized choices after the convolutional and pooling layers. These layers arrange connections between each neuron in a layer and every completely different layer’s neuron, enabling the neighborhood to know the relationships between choices extracted from the enter images. Inside the closing phases of neighborhood improvement, completely linked layers are generally used to generate the required output, equal to class probabilities in image classification duties.
Softmax Layer
Often, a softmax layer is inserted on the end of the neighborhood to remodel the class probabilities from the raw output scores in classification duties. To guarantee that the output scores add as a lot as one and may very well be understood as probabilities, the softmax carry out normalizes the values for each class. In consequence, the neighborhood can choose the class with the perfect likelihood to make predictions.
CNNs leverage convolutional layers with learnable filters to extract hierarchical choices from enter images, adopted by activation options to introduce non-linearity, pooling layers to downsample perform maps, completely linked layers for high-level perform illustration, and a softmax layer for classification duties. This construction permits CNNs to hold out good in different image-related duties, along with image classification, object detection, and segmentation.
Permit us to now apply the concepts to the dataset from the Analytics Vidhya hackathon.
Implementation of CNN
We’ll execute CNN implementation every with and with out change finding out. To begin, let’s first cope with the implementation with out change finding out.
Proper right here’s the step-by-step implementation:
Step1: Importing Libraries and Dependencies
As everyone knows , the very first step is to place in all wanted libraries and dependencies:
import pandas as pd
import numpy as np
import cv2
import seaborn as sns
import tensorflow as tf
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
from tensorflow.keras.fashions import Sequential
from tensorflow.math import confusion_matrix
from tensorflow.keras.callbacks import ModelCheckpoint
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, InputLayer
from glob import glob
from skimage.rework import resize
from keras.utils import to_categorical
from keras.fashions import Sequential
import keras
from keras.layers import Dense, Conv2D, MaxPool2D , Flatten
from tensorflow.keras import fashions, layers
Step2: Load the Dataset
information = pd.read_csv('/kaggle/enter/shipdataset/put together.csv')
Step3: Data Analysis
Now, let’s conduct some information analysis:
information.groupby('class').rely()
It’ll current insights into the distribution of lessons all through the dataset.
Step4: Visualization
Let’s now visualize this:
ship_categories = {1: 'Cargo', 2: 'Navy', 3: 'Service', 4: 'Cruise', 5: 'Tanker'}
information['category_mapped'] = information['category'].map(ship_categories)
sns.countplot(x='category_mapped', information=information)
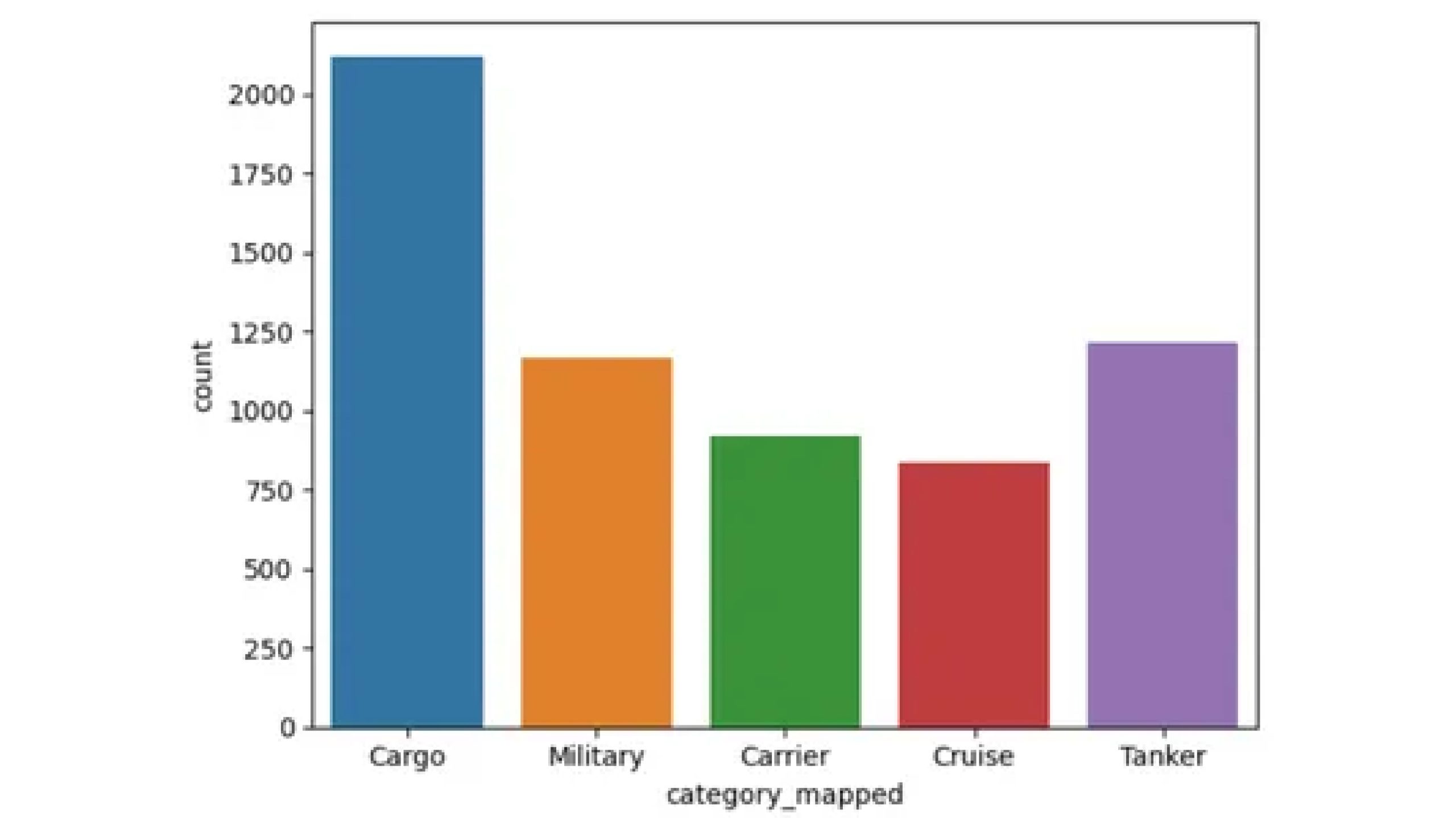
The countplot reveals that the dataset incorporates 2120 images categorized as Cargo, 1167 as Navy, 916 as Service, 832 as Cruise, and 1217 as Tanker.
Step5: Preprocessing the data
Now let’s preprocess the data with the help of code beneath:
X=[]
import cv2
for img_name in information.image:
img=cv2.imread('/kaggle/enter/shipdataset/images/'+img_name)
img_resized = cv2.resize(img, (224, 224))
X.append(img_resized)
X=np.array(X)
This code lots of images from a list, resizes them to 224×224 pixels using OpenCV, and outlets the resized images in a NumPy array.
Step6: Plotting
Now let’s plot them after resizing.
nrow = 5
ncol = 4
fig1 = plt.decide(figsize=(15, 15))
fig1.suptitle('After Resizing', measurement=32)
for i in range(20):
plt.subplot(nrow, ncol, i + 1)
plt.imshow(X[i])
plt.title('class = {x}, Ship = {y}'.format(x=information["category"][i],
y=ship_categories[data["category"][i]]))
plt.axis('Off')
plt.grid(False)
plt.current()
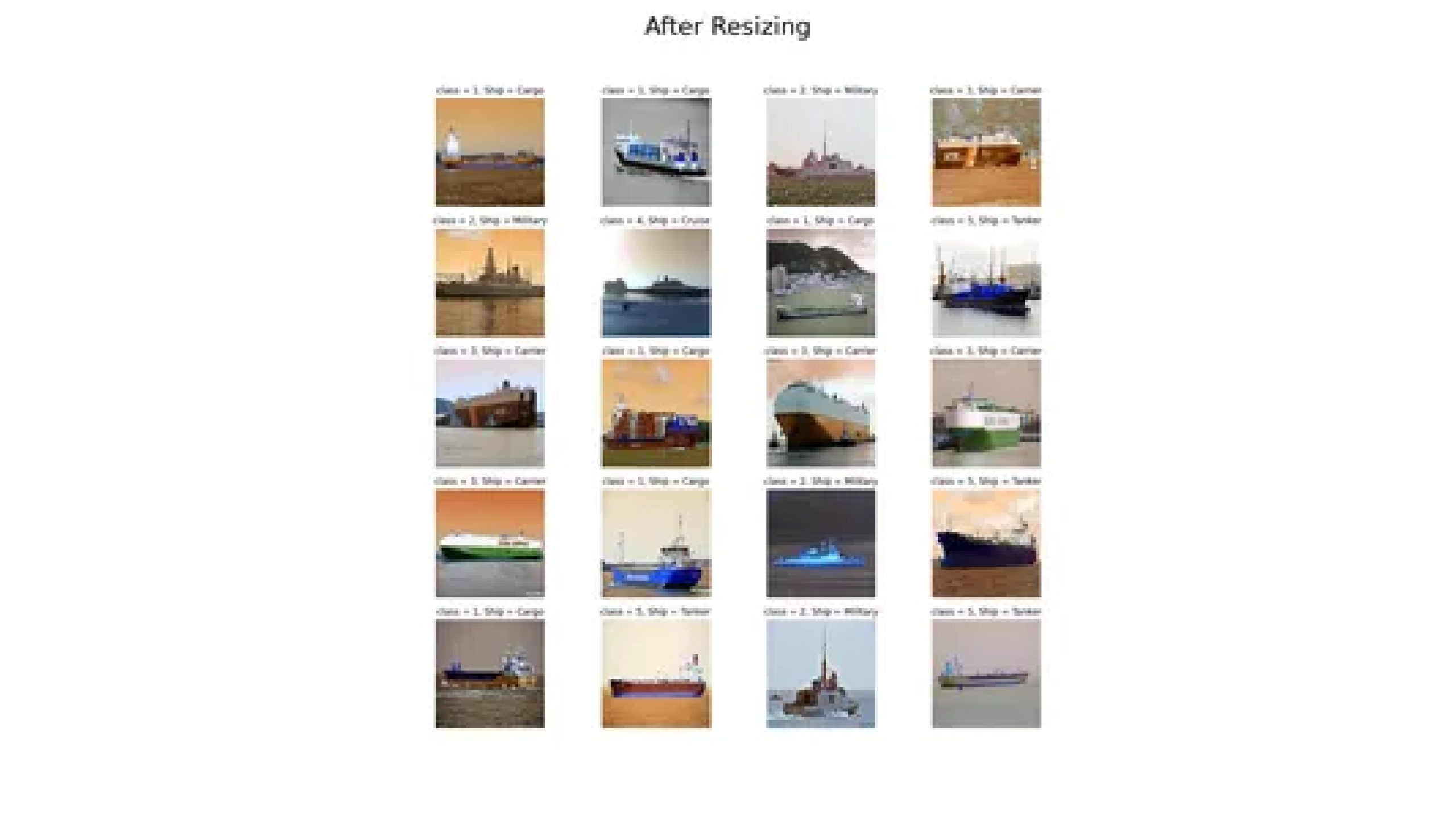
y=information.class.values
y=y-1
This step subtracts 1 from each price throughout the information.class array, storing the consequence throughout the variable y.
The purpose of this operation could very properly be to control the category labels. It’s widespread in machine finding out duties to begin out indexing from 0 as an alternative of 1, significantly when dealing with classification duties. To align the labels with zero-based indexing, subtracting 1 from the category labels is usually achieved, as required by machine finding out algorithms or libraries.
X = X.astype('float32') / 255
y = to_categorical(y)
This code converts pixel values in X to floats between 0 and 1 and one-hot encodes categorical labels in y.
Step7: Data Splitting into Put together/Verify Dataset
Break up the dataset into teaching and testing models using the train_test_split carry out.
X_train, X_test, y_train, y_test = train_test_split(X, y,
test_size=0.2, random_state=42)
Defining CNN Model: Define a CNN model using TensorFlow’s Sequential API, specifying the convolutional and pooling layers.
CNN_model = fashions.Sequential([
layers.Conv2D(64, (3, 3), activation='relu', padding='same',
input_shape=(224, 224, 3)),
layers.Conv2D(64, (3, 3), padding='same', activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(128, (3, 3), padding='same', activation='relu'),
layers.Conv2D(128, (3, 3), padding='same', activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.GlobalAveragePooling2D(),
layers.Dense(128, activation='relu'),
layers.Dense(128, activation='relu'),
layers.Dense(128, activation='relu'),
layers.Dense(5, activation='softmax')
])
CNN_model.summary()
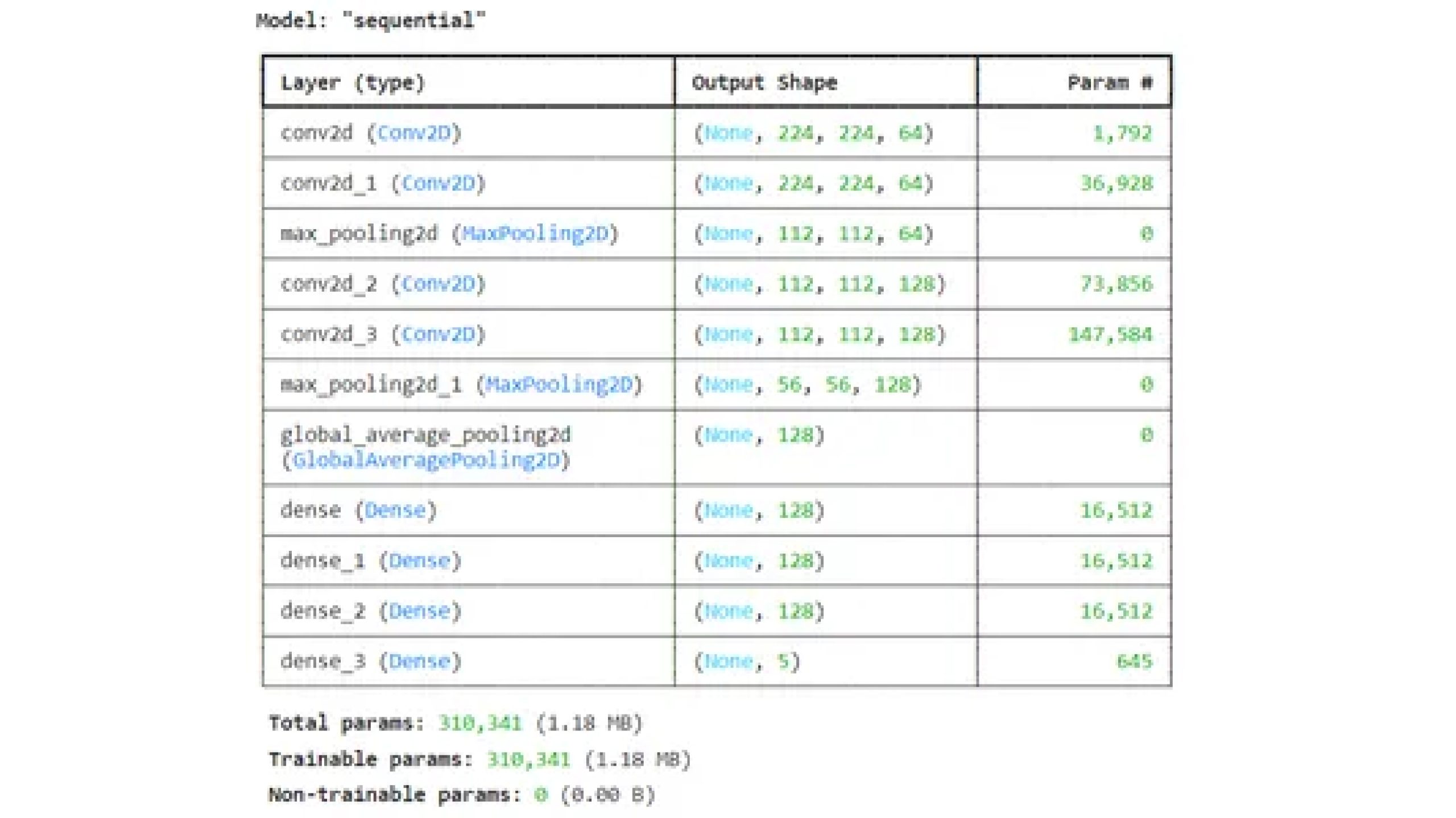
Step8: Model Teaching
rain the CNN model on the teaching information, establishing early stopping and model checkpoint to forestall overfitting and save the simplest model.
Compile the model with adam optimizer and loss as categorical cross entropy as a result of it’s multiclass classification
from tensorflow.keras.optimizers import Adam
model.compile(optimizer="adam",
loss="categorical_crossentropy",
metrics=['accuracy',tf.keras.metrics.F1Score()])
Saving the simplest model on validation loss
from tensorflow.keras.callbacks import EarlyStopping, ModelCheckpoint
early_stop = EarlyStopping(monitor="val_loss",
persistence=3, restore_best_weights=True)
checkpoint = ModelCheckpoint('best_model.keras',
monitor="val_loss", save_best_only=True, mode="min")
Step9: Changing into the Model
historic previous = model.match(X_train, y_train,
epochs=20,
batch_size=32,
validation_data=(X_test, y_test),
callbacks=[early_stop, checkpoint])
Step10: Model Evaluation
Now let’s do model evaluation using educated model.
from sklearn.metrics import f1_score
y_pred = model.predict(X_test)
Altering predictions from one-hot encoded format to class labels.
y_pred_labels = np.argmax(y_pred, axis=1)
y_true_labels = np.argmax(y_test, axis=1)
from sklearn.metrics import classification_report
report = classification_report(y_true_labels, y_pred_labels)
print("Classification Report:")
print(report)
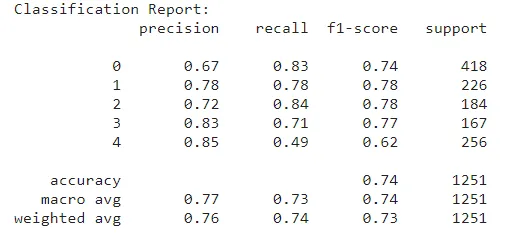
Plotting teaching & validation loss values
plt.decide(figsize=(10, 6))
plt.plot(historic previous.historic previous['loss'], label="Teaching Loss")
plt.plot(historic previous.historic previous['val_loss'], label="Validation Loss")
plt.title('Model Loss')
plt.xlabel('Epoch')
plt.ylabel('Loss')
plt.legend()
plt.grid(True)
plt.current()
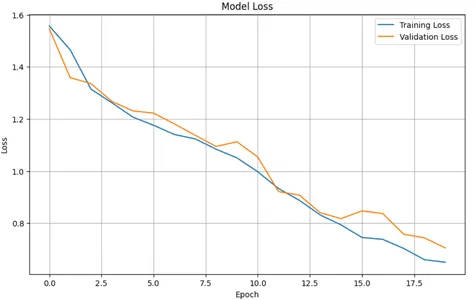
import matplotlib.pyplot as plt
plt.plot(historic previous.historic previous['accuracy'], label="Teaching accuracy")
plt.plot(historic previous.historic previous['val_accuracy'], label="Validation accuracy")
plt.title('Teaching and Validation accuracy')
plt.xlabel('Epoch')
plt.ylabel('Accuracy')
plt.legend()
plt.grid(True)
plt.current()
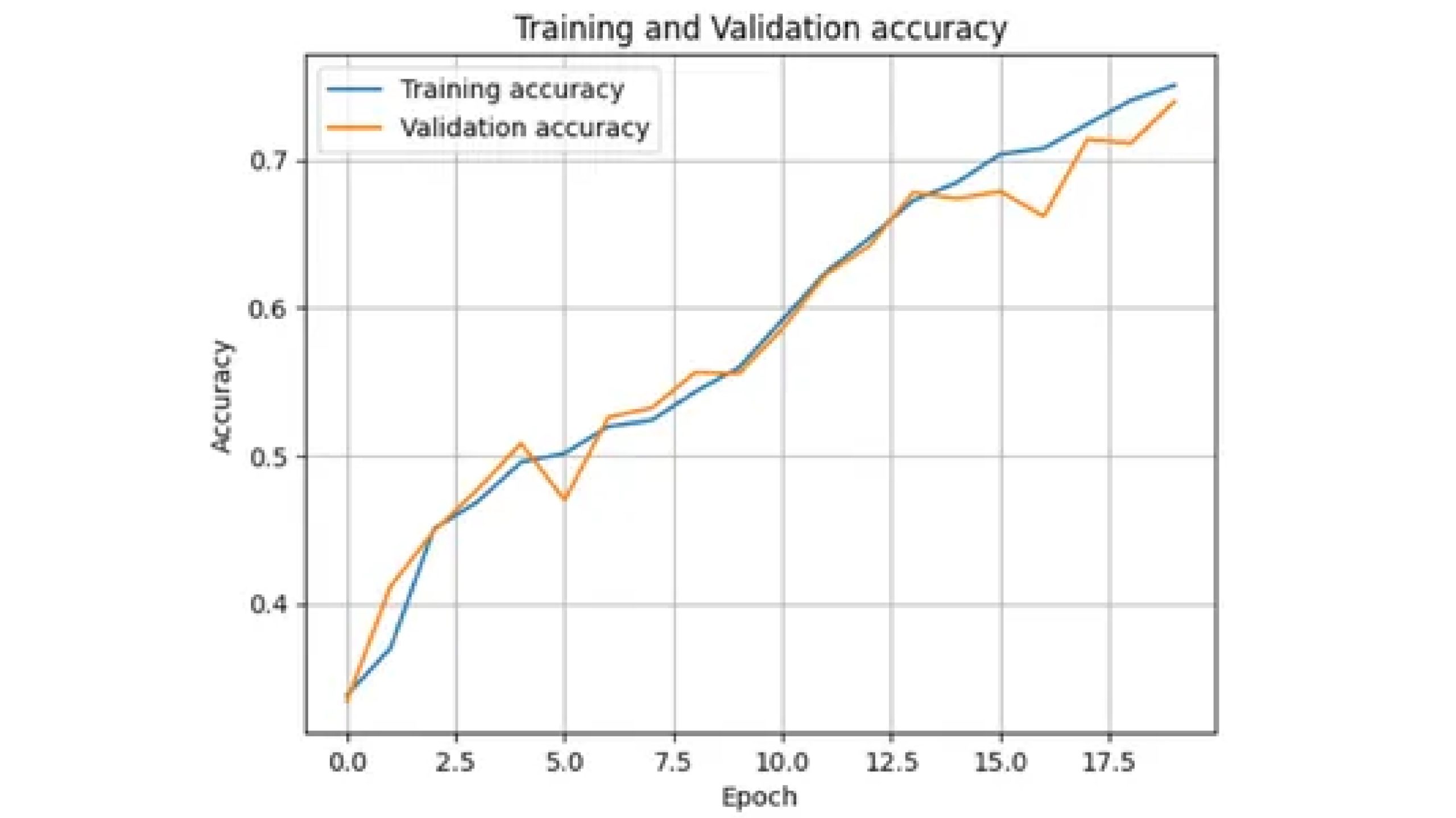
Testing of Data
Preparing and preprocessing the check out information equally to the teaching information, make predictions using the educated model, and visualize some sample predictions along with their predicted classes.
check out=pd.read_csv('/kaggle/enter/test-data/test_ApKoW4T.csv')
X_test=[]
import cv2
for img_name in check out.image:
img=cv2.imread('/kaggle/enter/shipdataset/images/'+img_name)
img_resized = cv2.resize(img, (224, 224))
X_test.append(img_resized)
X_test=np.array(X_test)
X_test = X_test.astype('float32') / 255
Making Prediction
predictions=model.predict(X_test)
predicted_class= np.argmax(predictions,axis=1)
predicted_class=predicted_class+1
csv_=check out.copy()
csv_
csv_['category']=predicted_class
csv_.head()
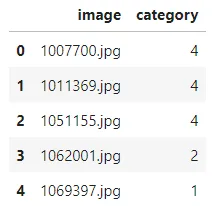
csv_['category'].value_counts()
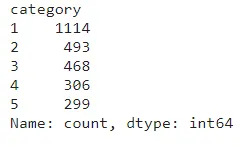
Save Predictions in CSV
csv_.to_csv('prediction1.csv',index=False)
Plotting the Predicted Verify Data
plt.decide(figsize=(8, 8))
for i in range(20):
plt.subplot(4, 5, i + 1)
plt.imshow(X_test[i])
plt.title(f'Predicted Class: {ship_categories[predicted_class[i]]}', fontsize=8)
plt.tight_layout()
plt.savefig('prediction_plot1.png')
plt.current()
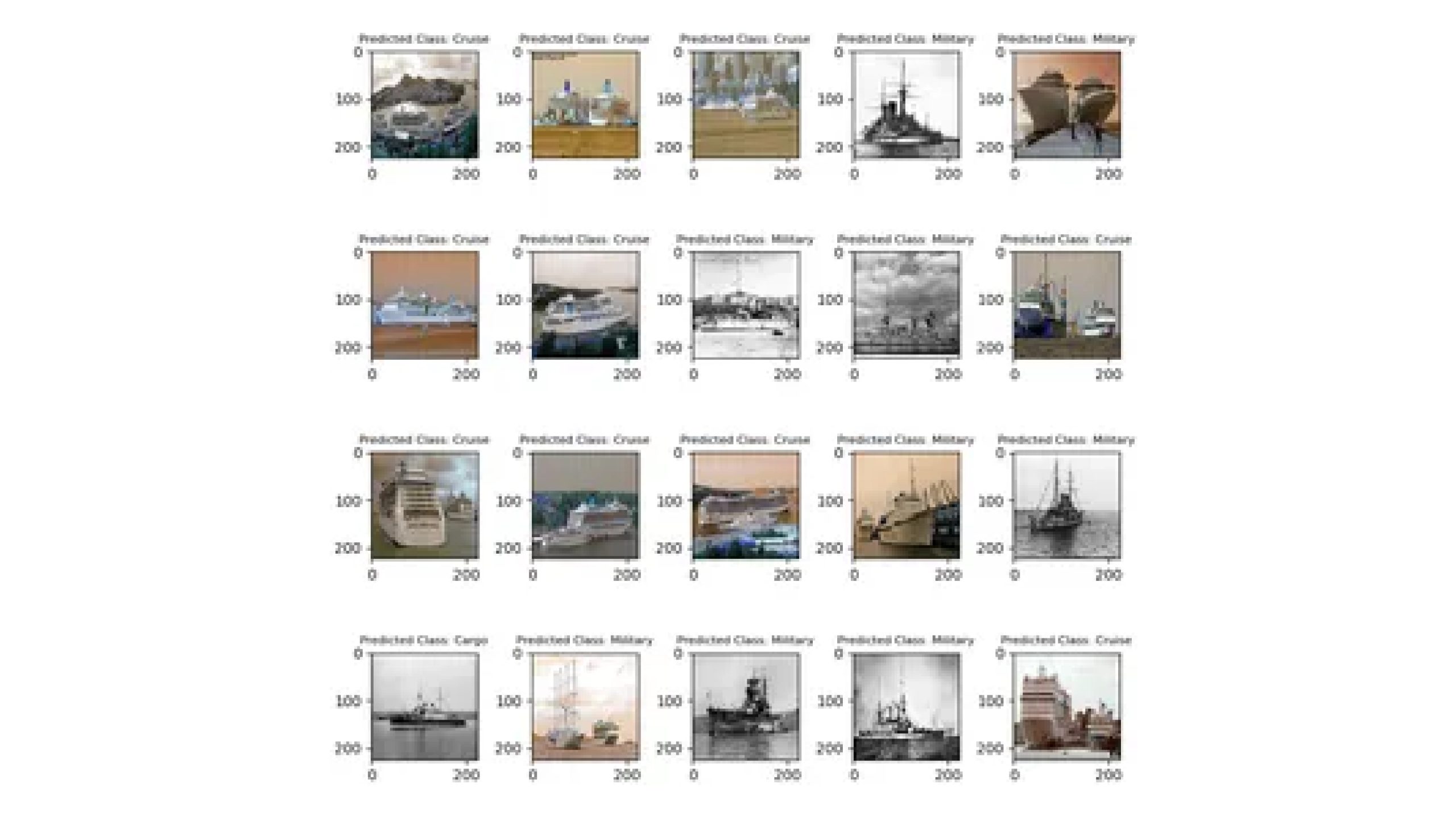
Now let’s use change finding out to unravel this downside for this we are going to seemingly be using resnet.
Understanding Mobilenet
Mobilenet is a sort of convolutional neural neighborhood (CNN) designed significantly for cell and embedded models . It’s recognized for being atmosphere pleasant and lightweight, making it splendid for circumstances the place processing power and battery life are restricted.
Proper right here’s a breakdown of Mobilenet’s key choices:
- Effectivity: Mobilenet employs depthwise separable convolutions, which divide information processing into two steps: depthwise convolution using a single filter for each enter channel and pointwise convolution using 1×1 filters.
- Lightweight: Mobilenet lowers the amount of parameters wished by the model by minimizing computations. Due to this the model will seemingly be smaller, which is important for cell models with constrained storage.
- Capabilities: It is useful for various duties on cell models, along with image classification, object detection, and facial recognition.
You probably can study further about MobileNet by clicking proper right here.
We’re going to benefit from MobileNet for this exercise. All of the issues stays fixed from importing libraries to information splitting(an identical step as with out change finding out) . Furthermore, we have now to import the MobileNet library.
from keras.capabilities import MobileNet
from keras.fashions import Model
from keras.layers import Dense, GlobalAveragePooling2D
from keras.layers import Dropout, BatchNormalization
Loading Pre-trained Model
Now load the Pre-trained Model as base model
base_model = MobileNet(weights="imagenet", include_top=False)
Freeze all layers throughout the base model:
for layer in base_model.layers:
layer.trainable = False
Assemble Model Using Purposeful Function
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(1024, activation='relu')(x)
x = BatchNormalization()(x)
x = Dropout(0.5)(x) # Add dropout with a worth of 0.5
predictions = Dense(5, activation='softmax')(x)
#Creating the model
model = Model(inputs=base_model.enter, outputs=predictions)
Compiling the Model
Compile the model with adam optimizer and loss as categorical cross entropy as a result of it’s multiclass classification
from tensorflow.keras.optimizers import Adam
model.compile(optimizer="adam",
loss="categorical_crossentropy",
metrics=['accuracy',tf.keras.metrics.F1Score()])
Saving the Biggest Model on Validation Loss
from tensorflow.keras.callbacks import EarlyStopping, ModelCheckpoint
early_stop = EarlyStopping(monitor="val_loss", persistence=2, restore_best_weights=True)
checkpoint = ModelCheckpoint('best_model.keras', monitor="val_loss",
save_best_only=True, mode="min")
Changing into the Model
historic previous = model.match(X_train, y_train,
epochs=20,
batch_size=32,
validation_data=(X_test, y_test),
callbacks=[early_stop,checkpoint])
Model Evaluation
Now let’s do model evaluation.
from sklearn.metrics import f1_score
#Making predictions using the educated model
y_pred = model.predict(X_test)
#Altering predictions from one-hot encoded format to class labels
y_pred_labels = np.argmax(y_pred, axis=1)
y_true_labels = np.argmax(y_test, axis=1)
from sklearn.metrics import classification_report
report = classification_report(y_true_labels, y_pred_labels)
print("Classification Report:")
print(report)
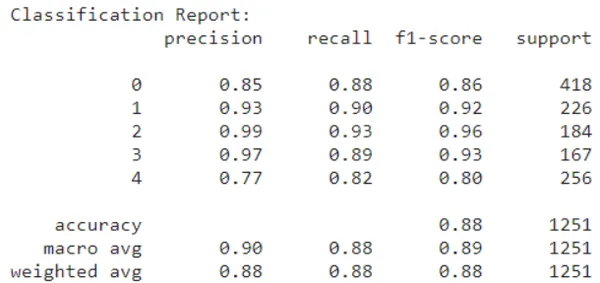
Plotting Teaching and Validation Loss Values
plt.decide(figsize=(10, 6))
plt.plot(historic previous.historic previous['loss'], label="Teaching Loss")
plt.plot(historic previous.historic previous['val_loss'], label="Validation Loss")
plt.title('Model Loss')
plt.xlabel('Epoch')
plt.ylabel('Loss')
plt.legend()
plt.grid(True)
plt.current()
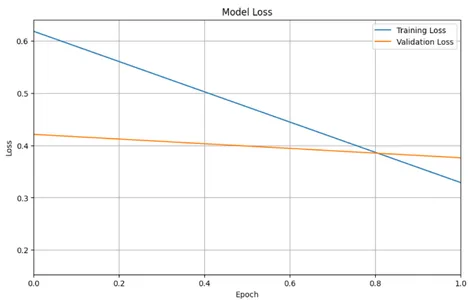
Plotting Accuracy Curve
import matplotlib.pyplot as plt
plt.plot(historic previous.historic previous['accuracy'], label="Teaching accuracy")
plt.plot(historic previous.historic previous['val_accuracy'], label="Validation accuracy")
plt.title('Teaching and Validation accuracy')
plt.xlabel('Epoch')
plt.ylabel('Accuracy')
plt.legend()
plt.grid(True)
plt.current()
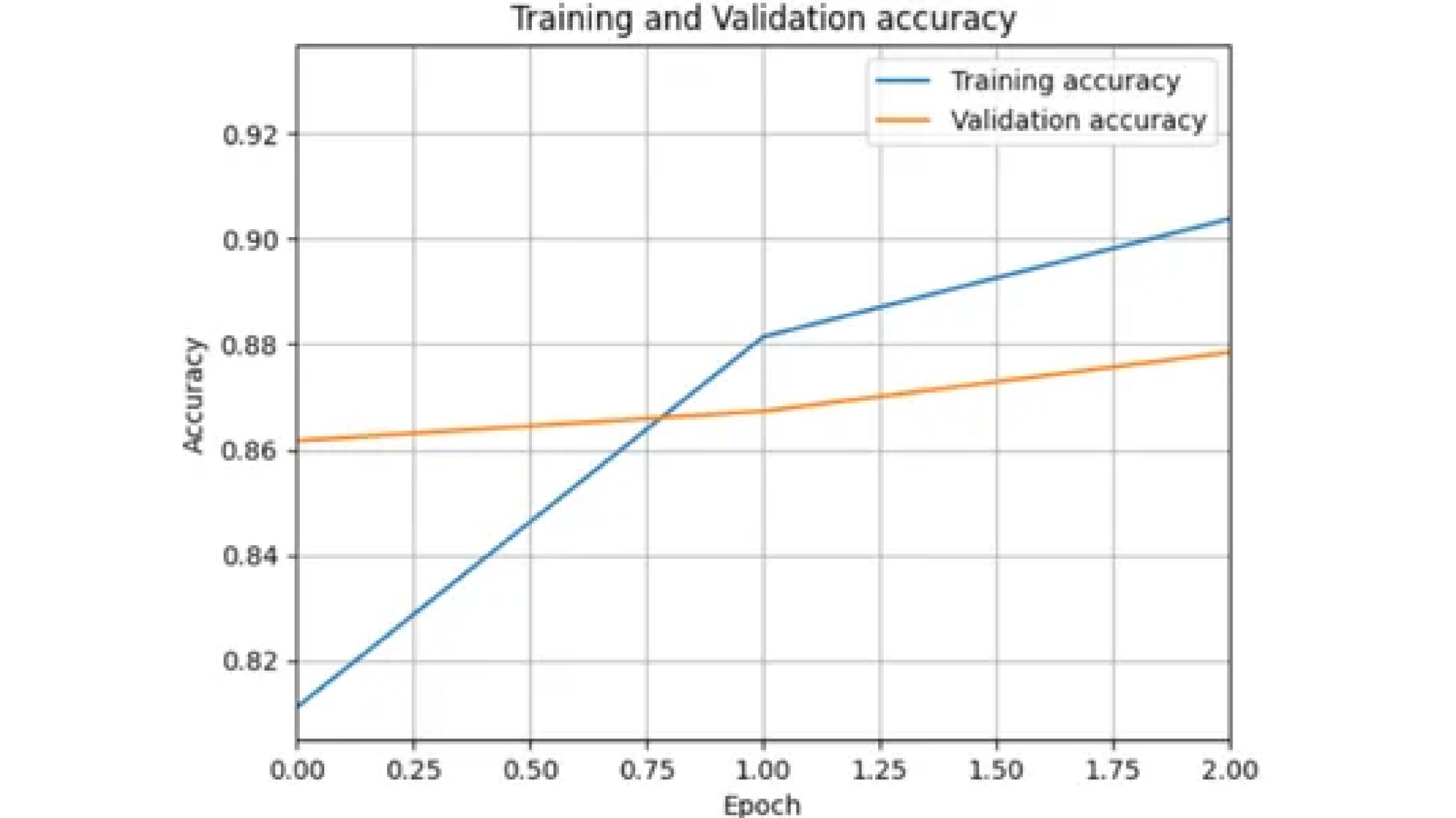
Now do the prediction on check out information an identical as achieved in with out change finding out
Conclusion
This study explores two approaches to ship classification using Convolutional Neural Networks (CNNs). The first entails setting up a CNN from scratch with out change finding out strategies, whereas the second makes use of change finding out using MobileNet construction. Every methods current potential choices for ship classification, with change finding out offering greater effectivity with a lot much less teaching information. The choice will rely on computational sources, dataset measurement, and desired effectivity metrics.
Incessantly Requested Questions
A. OpenCV is a powerful instrument for image processing that provides a wide range of options for duties equal to image manipulation, perform extraction, and object detection. It presents different functionalities to preprocess raw image information, extract associated choices, and enhance image top quality.
A. CNNs excel at finding out hierarchical representations of images, robotically extracting choices at fully completely different ranges of abstraction. They embody a lot of layers, along with convolutional layers, activation options, pooling layers, completely linked layers, and softmax layers, which work collectively to course of and extract choices from enter images.
A. In change finding out, a model educated on one exercise serves as the beginning line for a model on a second exercise. Inside the context of neural networks, change finding out entails taking a pre-trained model (usually educated on a giant dataset) and fine-tuning it for a selected exercise or dataset. This technique might also assist improve model effectivity, significantly when the model new dataset is small or similar to the distinctive dataset.