Introduction
Kolmogorov-Arnold Networks, additionally known as KAN, are the most recent improvement in neural networks. Primarily based totally on the Kolgomorov-Arnold illustration theorem, they’ve the potential to be a viable completely different to Multilayer Perceptrons (MLP). In distinction to MLPs with mounted activation options at each node, KANs use learnable activation options on edges, altering linear weights with univariate options as parameterized splines.
A evaluation group from the Massachusetts Institute of Know-how, California Institute of Know-how, Northeastern School, and The NSF Institute for Artificial Intelligence and Elementary Interactions provided Kolmogorov-Arnold Networks (KANs) as a promising different for MLPs in a present paper titled “KAN: Kolmogorov-Arnold Networks.”
Learning Goals
- Be taught and understand a model new form of neural group known as Kolmogorov-Arnold Group which will current accuracy and interpretability.
- Implement Kolmogorov-Arnold Networks using Python libraries.
- Understand the variations between Multi-Layer Perceptrons and Kolmogorov-Arnold Networks.
This textual content was printed as a part of the Data Science Blogathon.
Kolmogorov-Arnold representation theorem
According to the Kolmogorov-Arnold representation theorem, any multivariate continuous function can be defined as:
Here:
ϕqp : [0, 1] → R and Φq : R → R
Any multivariate function can be expressed as a sum of univariate functions and additions. This might make you think machine learning can become easier by learning high-dimensional functions through simple one-dimensional ones. However, since univariate functions can be non-smooth, this theorem was considered theoretical and impossible in practice. However, the researchers of KAN realized the potential of this theorem by expanding the function to greater than 2n+1 layers and for real-world, smooth functions.
What are Multi-layer Perceptrons?
These are the simplest forms of ANNs, the place information flows in a single course, from enter to output. The group construction would not have cycles or loops. Multilayer perceptrons (MLP) are a form of feedforward neural group.
Multilayer Perceptrons are a form of feedforward neural group. Feedforward Neural Networks are simple artificial neural networks whereby information strikes forward, in a single course, from enter to output by way of a hidden layer.
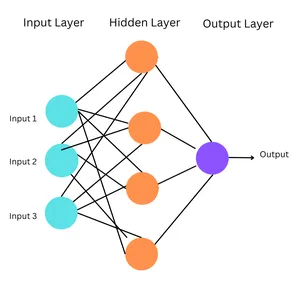
Working of MLPs
- Enter Layer: The enter layer consists of nodes representing the enter info’s choices. Each node corresponds to 1 operate.
- Hidden Layers: MLPs have plenty of hidden layers between the enter and output layers. The hidden layers enable the group to be taught superior patterns and relationships throughout the info.
- Output Layer: The output layer produces the final word predictions or classifications.
- Connections and Weights: Each connection between neurons in adjoining layers is expounded to a weight, determining its vitality. All through teaching, these weights are adjusted by backpropagation, the place the group learns to attenuate the excellence between its predictions and the exact purpose values.
- Activation Options: Each neuron (moreover these throughout the enter layer) applies an activation function to the weighted sum of its inputs. This introduces non-linearity into the group.
Simplified Elements

Proper right here:
- σ = activation function
- W = tunable weights that symbolize connection strengths
- x = enter
- B = bias
MLPs are based totally on the widespread approximation theorem, which states {{that a}} feedforward neural group with a single hidden layer with a finite number of neurons can approximate any regular function on a compact subset as long as the function should not be a polynomial. This permits neural networks, significantly these with hidden layers, to represent quite a lot of superior options. Thus, MLPs are designed based totally on this (with plenty of hidden layers) to grab the intricate patterns in info. MLPs have mounted activation options on each node.
However, MLPs have plenty of drawbacks. MLPs in transformers take advantage of the model’s parameters, even these that are not related to the embedding layers. They’re moreover a lot much less interpretable. That’s how KANs come into the picture.
Kolmogorov-Arnold Networks (KANs)
A Kolmogorov-Arnold Group is a neural group with learnable activation options. At each node, the group learns the activation function. In distinction to MLPs with mounted node activation options, KANs have learnable activation options on edges. They modify the linear weights with parametrized splines.
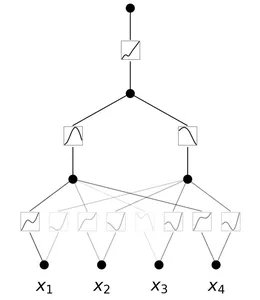
Advantages of KANs
Listed beneath are the advantages of KANs:
- Higher Flexibility: KANs are extraordinarily versatile attributable to their activation options and model construction, thus allowing larger illustration of superior info.
- Adaptable Activation Options: In distinction to in MLPs, the activation options in KANs aren’t mounted. Since their activation options are learnable on edges, they will adapt and regulate to utterly completely different info patterns, thus efficiently capturing quite a few relationships.
- Increased Complexity Coping with: They modify the linear weights in MLPs by parametrized splines, thus they will take care of superior, non-linear info.
- Superior Accuracy: KANs have demonstrated larger accuracy in coping with high-dimensional info
- Extraordinarily Interpretable: They reveal the buildings and topological relationships between the information thus they will merely be interpreted.
- Numerous Functions: they will perform various duties like regression, partial differential equations fixing, and steady finding out.
Moreover study: Multi-Layer Perceptrons: Notations and Trainable Parameters
Simple Implementation of KANs
Let’s implement KANs with the help of a simple occasion. We will create a personalized dataset of the function: f(x, y) = exp(cos(pi*x) + y^2). This function takes two inputs, calculates the cosine of pi*x, supplies the sq. of y to it, after which calculates the exponential of the consequence.
Requirements of Python library mannequin:
- Python==3.9.7
- matplotlib==3.6.2
- numpy==1.24.4
- scikit_learn==1.1.3
- torch==2.2.2
!pip arrange git+https://github.com/KindXiaoming/pykan.git
import torch
import numpy as np
##create a dataset
def create_dataset(f, n_var=2, n_samples=1000, split_ratio=0.8):
# Generate random enter info
X = torch.rand(n_samples, n_var)
# Compute the purpose values
y = f(X)
# Lower up into teaching and examine models
split_idx = int(n_samples * split_ratio)
train_input, test_input = X[:split_idx], X[split_idx:]
train_label, test_label = y[:split_idx], y[split_idx:]
return {
'train_input': train_input,
'train_label': train_label,
'test_input': test_input,
'test_label': test_label
}
# Define the model new function f(x, y) = exp(cos(pi*x) + y^2)
f = lambda x: torch.exp(torch.cos(torch.pi*x[:, [0]]) + x[:, [1]]**2)
dataset = create_dataset(f, n_var=2)
print(dataset['train_input'].type, dataset['train_label'].type)
##output: torch.Dimension([800, 2]) torch.Dimension([800, 1])
from kan import *
# create a KAN: 2D inputs, 1D output, and 5 hidden neurons.
# cubic spline (okay=3), 5 grid intervals (grid=5).
model = KAN(width=[2,5,1], grid=5, okay=3, seed=0)
# plot KAN at initialization
model(dataset['train_input']);
model.plot(beta=100)
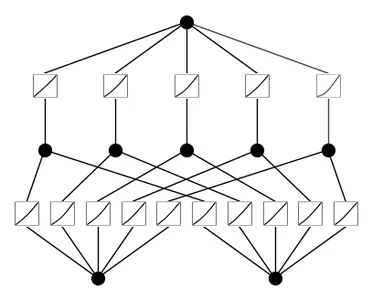
## observe the model
model.observe(dataset, select="LBFGS", steps=20, lamb=0.01, lamb_entropy=10.)
## output: observe loss: 7.23e-02 | examine loss: 8.59e-02
## output: | reg: 3.16e+01 : 100%|██| 20/20 [00:11<00:00, 1.69it/s]
model.plot()
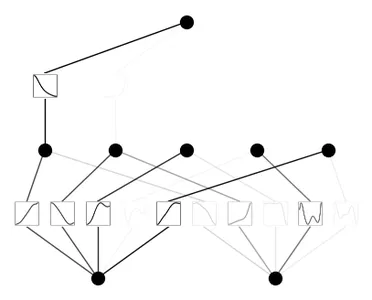
model.prune()
model.plot(masks=True)
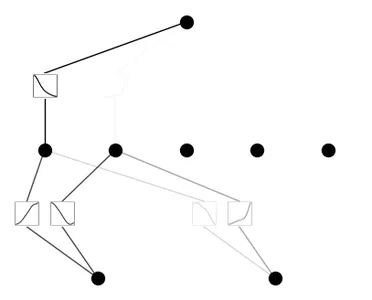
model = model.prune()
model(dataset['train_input'])
model.plot()
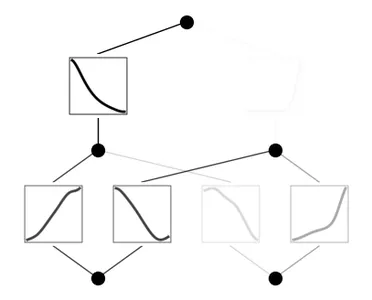
model.observe(dataset, select="LBFGS", steps=100)
model.plot()
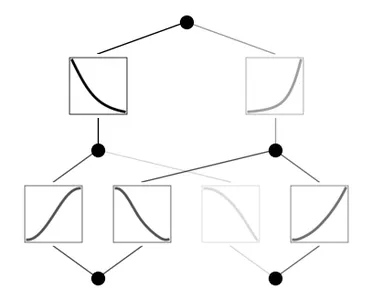
Code Clarification
- Arrange the Pykan library from Git Hub.
- Import libraries.
- The create_dataset function generates random enter info (X) and computes the purpose values (y) using the function f. The dataset is then minimize up into teaching and examine models based totally on the minimize up ratio. The parameters of this function are:
- f: function to generate the purpose values.
- n_var: number of enter variables.
- n_samples: entire number of samples
- split_ratio: ratio to separate the dataset into teaching and examine models, and it returns a dictionary containing teaching and examine inputs and labels.
- Create a function of the form: f(x, y) = exp(cos(pi*x) + y^2)
- Identify the function create_dataset to create a dataset using the beforehand outlined function f with 2 enter variables.
- Print the type of teaching inputs and their labels.
- Initialize a KAN model with 2-dimensional inputs, 1-dimensional output, 5 hidden neurons, cubic spline (okay=3), and 5 grid intervals (grid=5)
- Plot the KAN model at initialization.
- Put together the KAN model using the equipped dataset for 20 steps using the LBFGS optimizer.
- After teaching, plot the expert model.
- Prune the model and plot the pruned model with the masked neurons.
- Prune the model as soon as extra, think about it on the teaching enter, and plot the pruned model.
- Re-train the pruned model for an additional 100 steps.
MLP vs KAN
MLP | KAN |
Fixed node activation options | Learnable activation options |
Linear weights | Parametrized splines |
A lot much less interpretable | Additional interpretable |
A lot much less versatile and adaptable as compared with KANs | Extraordinarily versatile and adaptable |
Sooner teaching time | Slower teaching time |
Primarily based totally on Frequent Approximation Theorem | Primarily based totally on Kolmogorov-Arnold Illustration Theorem |
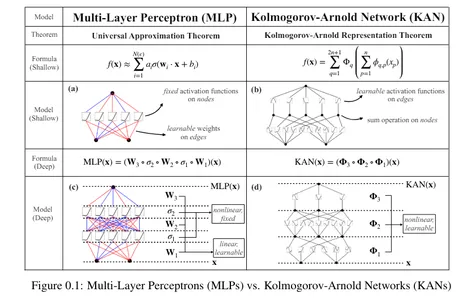
Conclusion
The invention of KANs signifies a step in route of advancing deep finding out methods. By providing larger interpretability and accuracy than MLPs, they may very well be a extra good selection when interpretability and accuracy of the outcomes are the first purpose. However, MLPs may very well be a additional smart decision for duties the place tempo is necessary. Evaluation is consistently occurring to boost these networks, however for now, KANs symbolize an thrilling completely different to MLPs.
The media confirmed on this text won’t be owned by Analytics Vidhya and is used on the Author’s discretion.
Frequently Asked Questions
A. Ziming Liu, Yixuan Wang, Sachin Vaidya, Fabian Ruehle, James Halverson, Marin Soljaci, Thomas Y. Hou, Max Tegmark are the researchers involved in the dQevelopment of KANs.
A. Fixed activation functions are mathematical functions applied to the outputs of neurons in neural networks. These functions remain constant throughout training and are not updated or adjusted based on the network’s learning. Ex: Sigmoid, tanh, ReLU.
Learnable activation functions are adaptive and modified during the training process. Instead of being predefined, they are updated through backpropagation, allowing the network to learn the most suitable activation functions.
A. One limitation of KANs is their slower training time due to their complex architecture. They require more computations during the training process since they replace the linear weights with spline-based functions that require additional computations to learn and optimize.
A. If your task requires more accuracy and interpretability and training time isn’t limited, you can proceed with KANs. If training time is critical, MLPs are a practical option.
A. The LBFGS optimizer stands for “Limited-memory Broyden–Fletcher–Goldfarb–Shanno” optimizer. It is a popular algorithm for parameter estimation in machine learning and numerical optimization.