Introduction
Immediate engineering has turn out to be pivotal in leveraging Massive Language fashions (LLMs) for various purposes. As you all know, primary immediate engineering covers elementary methods. Nevertheless, advancing to extra subtle strategies permits us to create extremely efficient, context-aware, and strong language fashions. This text will delve into a number of superior immediate engineering methods utilizing LangChain. I’ve added code examples and sensible insights for builders.
In superior immediate engineering, we craft complicated prompts and use LangChain’s capabilities to construct clever, context-aware purposes. This consists of dynamic prompting, context-aware prompts, meta-prompting, and utilizing reminiscence to take care of state throughout interactions. These methods can considerably improve the efficiency and reliability of LLM-powered applications.
Studying Targets
- Study to create multi-step prompts that information the mannequin by way of complicated reasoning and workflows.
- Discover superior immediate engineering methods to regulate prompts primarily based on real-time context and person interactions for adaptive purposes.
- Develop prompts that evolve with the dialog or activity to take care of relevance and coherence.
- Generate and refine prompts autonomously utilizing the mannequin’s inner state and suggestions mechanisms.
- Implement reminiscence mechanisms to take care of context and data throughout interactions for coherent purposes.
- Use superior immediate engineering in real-world purposes like schooling, help, artistic writing, and analysis.
This text was printed as part of the Data Science Blogathon.
Setting Up LangChain
Be sure to arrange LangChain appropriately. A strong setup and familiarity with the framework are essential for superior purposes. I hope you all know learn how to arrange LangChain in Python.
Set up
First, set up LangChain utilizing pip:
pip set up langchain
Fundamental setup
from langchain import LangChain
from langchain.fashions import OpenAI
# Initialize the LangChain framework
lc = LangChain()
# Initialize the OpenAI mannequin
mannequin = OpenAI(api_key='your_openai_api_key')
Superior Immediate Structuring
Advanced prompt structuring is a complicated model that goes past easy directions or contextual prompts. It includes creating multi-step prompts that information the mannequin by way of logical steps. This system is crucial for duties that require detailed explanations, step-by-step reasoning, or complicated workflows. By breaking the duty into smaller, manageable parts, superior immediate structuring may help improve the mannequin’s potential to generate coherent, correct, and contextually related responses.
Functions of Superior Immediate Structuring
- Academic Instruments: Superior immediate engineering instruments can create detailed instructional content material, akin to step-by-step tutorials, complete explanations of complicated subjects, and interactive studying modules.
- Technical Assist:It may possibly assist present detailed technical help, troubleshooting steps, and diagnostic procedures for numerous techniques and purposes.
- Artistic Writing: In artistic domains, superior immediate engineering may help generate intricate story plots, character developments, and thematic explorations by guiding the mannequin by way of a sequence of narrative-building steps.
- Analysis Help: For analysis functions, structured prompts can help in literature evaluations, knowledge evaluation, and the synthesis of knowledge from a number of sources, guaranteeing an intensive and systematic manner.
Key Parts of Superior Immediate Structuring
Listed below are superior prompt engineering structuring:
- Step-by-Step Directions: By offering the mannequin with a transparent sequence of steps to observe, we are able to considerably enhance the standard of its output. That is significantly helpful for problem-solving, procedural explanations, and detailed descriptions. Every step ought to construct logically on the earlier one, guiding the mannequin by way of a structured thought course of.
- Intermediate Objectives: To assist make sure the mannequin stays on monitor, we are able to set intermediate targets or checkpoints inside the immediate. These targets act as mini-prompts inside the principle immediate, permitting the mannequin to concentrate on one facet of the duty at a time. This method might be significantly efficient in duties that contain a number of levels or require the mixing of varied items of knowledge.
- Contextual Hints and Clues: Incorporating contextual hints and clues inside the immediate may help the mannequin perceive the broader context of the duty. Examples embody offering background data, defining key phrases, or outlining the anticipated format of the response. Contextual clues be sure that the mannequin’s output is aligned with the person’s expectations and the particular necessities of the duty.
- Function Specification: Defining a selected position for the mannequin can improve its efficiency. As an illustration, asking the mannequin to behave as an skilled in a specific area (e.g., a mathematician, a historian, a medical physician) may help tailor its responses to the anticipated stage of experience and elegance. Function specification can enhance the mannequin’s potential to undertake totally different personas and adapt its language accordingly.
- Iterative Refinement: Superior immediate structuring usually includes an iterative course of the place the preliminary immediate is refined primarily based on the mannequin’s responses. This suggestions loop permits builders to fine-tune the immediate, making changes to enhance readability, coherence, and accuracy. Iterative refinement is essential for optimizing complicated prompts and attaining the specified output.
Instance: Multi-Step Reasoning
immediate = """
You're an skilled mathematician. Clear up the next downside step-by-step:
Drawback: If a automotive travels at a velocity of 60 km/h for two hours, how far does it journey?
Step 1: Determine the components to make use of.
Formulation: Distance = Pace * Time
Step 2: Substitute the values into the components.
Calculation: Distance = 60 km/h * 2 hours
Step 3: Carry out the multiplication.
Consequence: Distance = 120 km
Reply: The automotive travels 120 km.
"""
response = mannequin.generate(immediate)
print(response)
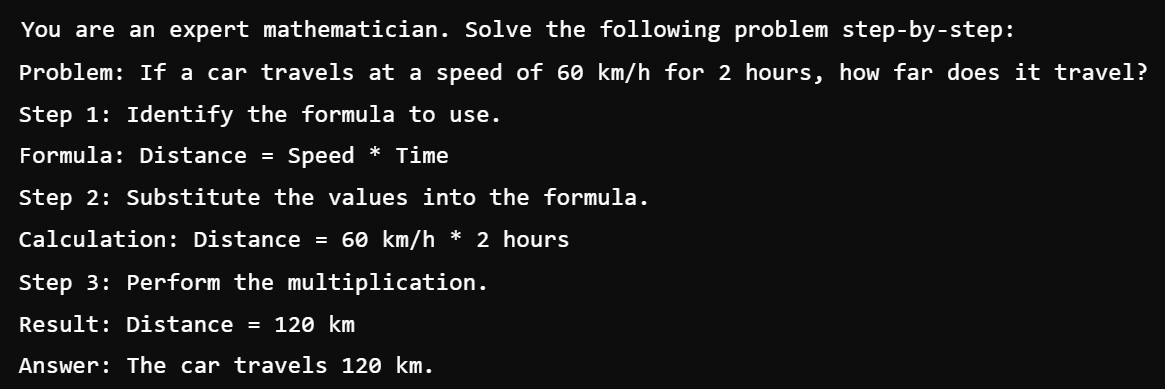
Dynamic Prompting
In Dynamic prompting, we regulate the immediate primarily based on the context or earlier interactions, enabling extra adaptive and responsive interactions with the language mannequin. Not like static prompts, which stay mounted all through the interplay, dynamic prompts can evolve primarily based on the evolving dialog or the particular necessities of the duty at hand. This flexibility in Dynamic prompting permits builders to create extra partaking, contextually related, and customized experiences for customers interacting with language fashions.
Functions of Dynamic Prompting
- Conversational Brokers: Dynamic prompting is crucial for constructing conversational brokers that may have interaction in pure, contextually related dialogues with customers, offering customized help and data retrieval.
- Interactive Studying Environments: In instructional sectors, dynamic prompting can improve interactive studying environments by adapting the educational content material to the learner’s progress and preferences and may present tailor-made suggestions and help.
- Data Retrieval Programs: Dynamic prompting can enhance the effectiveness of knowledge retrieval techniques by dynamically adjusting and updating the search queries primarily based on the person’s context and preferences, resulting in extra correct and related search outcomes.
- Personalised Suggestions: Dynamic prompting can energy customized advice techniques by dynamically producing prompts primarily based on person preferences and searching historical past. This method suggests related content material and merchandise to customers primarily based on their pursuits and previous interactions.
Strategies for Dynamic Prompting
- Contextual Question Growth: This includes increasing the preliminary immediate with further context gathered from the continued dialog or the person’s enter. This expanded immediate provides the mannequin a richer understanding of the present context, enabling extra knowledgeable and related responses.
- Person Intent Recognition: By analyzing the person’s intent and extracting the important thing data from their queries, builders can dynamically generate prompts that handle the particular wants and necessities expressed by the person. This may make sure the mannequin’s responses are tailor-made to the person’s intentions, resulting in extra satisfying interactions.
- Adaptive Immediate Era: Dynamic prompting can even generate prompts on the fly primarily based on the mannequin’s inner state and the present dialog historical past. These dynamically generated prompts can information the mannequin in the direction of producing coherent responses that align with the continued dialogue and the person’s expectations.
- Immediate Refinement by way of Suggestions: By including suggestions mechanisms into the prompting course of, builders can refine the immediate primarily based on the mannequin’s responses and the person’s suggestions. This iterative suggestions loop allows steady enchancment and adaptation, resulting in extra correct and efficient interactions over time.
Instance: Dynamic FAQ Generator
faqs = {
"What's LangChain?": "LangChain is a framework for constructing purposes powered by giant language fashions.",
"How do I set up LangChain?": "You possibly can set up LangChain utilizing pip: `pip set up langchain`."
}
def generate_prompt(query):
return f"""
You're a educated assistant. Reply the next query:
Query: {query}
"""
for query in faqs:
immediate = generate_prompt(query)
response = mannequin.generate(immediate)
print(f"Query: {query}nAnswer: {response}n")
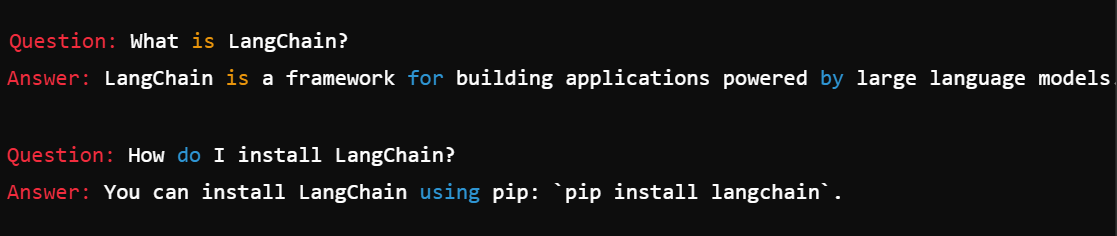
Context-Conscious Prompts
Context-aware prompts symbolize a classy method to partaking with language fashions. It includes the immediate to dynamically regulate primarily based on the context of the dialog or the duty at hand. Not like static prompts, which stay mounted all through the interplay, context-aware prompts evolve and adapt in actual time, enabling extra nuanced and related interactions with the mannequin. This system leverages the contextual data in the course of the interplay to information the mannequin’s responses. It helps in producing output that’s coherent, correct, and aligned with the person’s expectations.
Functions of Context-Conscious Prompts
- Conversational Assistants: Context-aware prompts are important for constructing conversational assistants to have interaction in pure, contextually related dialogues with customers, offering customized help and data retrieval.
- Process-Oriented Dialog Programs: In task-oriented dialog techniques, context-aware prompts allow the mannequin to grasp and reply to person queries within the context of the particular activity or area and information the dialog towards attaining the specified aim.
- Interactive Storytelling: Context-aware prompts can improve interactive storytelling experiences by adapting the narrative primarily based on the person’s selections and actions, guaranteeing a customized and immersive storytelling expertise.
- Buyer Assist Programs: Context-aware prompts can enhance the effectiveness of buyer help techniques by tailoring the responses to the person’s question and historic interactions, offering related and useful help.
Strategies for Context-Conscious Prompts
- Contextual Data Integration: Context-aware prompts take contextual data from the continued dialog, together with earlier messages, person intent, and related exterior knowledge sources. This contextual data enriches the immediate, giving the mannequin a deeper understanding of the dialog’s context and enabling extra knowledgeable responses.
- Contextual Immediate Growth: Context-aware prompts dynamically increase and adapt primarily based on the evolving dialog, including new data and adjusting the immediate’s construction as wanted. This flexibility permits the immediate to stay related and responsive all through the interplay and guides the mannequin towards producing coherent and contextually acceptable responses.
- Contextual Immediate Refinement: Because the dialog progresses, context-aware prompts might bear iterative refinement primarily based on suggestions from the mannequin’s responses and the person’s enter. This iterative course of permits builders to repeatedly regulate and optimize the immediate to make sure that it precisely captures the evolving context of the dialog.
- Multi-Flip Context Retention: Context-aware prompts keep a reminiscence of earlier interactions after which add this historic context to the immediate. This permits the mannequin to generate coherent responses with the continued dialogue and supply a dialog that’s extra up to date and coherent than a message.
Instance: Contextual Dialog
dialog = [
"User: Hi, who won the 2020 US presidential election?",
"AI: Joe Biden won the 2020 US presidential election.",
"User: What were his major campaign promises?"
]
context = "n".be a part of(dialog)
immediate = f"""
Proceed the dialog primarily based on the next context:
{context}
AI:
"""
response = mannequin.generate(immediate)
print(response)
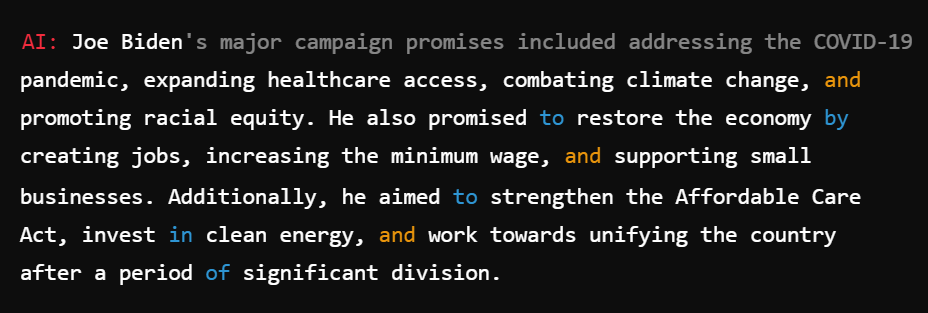
Meta-prompting is used to boost the sophistication and flexibility of language fashions. Not like standard prompts, which give specific directions or queries to the mannequin, meta-prompts function at the next stage of abstraction, which guides the mannequin in producing or refining prompts autonomously. This meta-level steering empowers the mannequin to regulate its prompting technique dynamically primarily based on the duty necessities, person interactions, and inner state. It ends in fostering a extra agile and responsive dialog.
Functions of Meta-Prompting
- Adaptive Immediate Engineering: Meta-prompting allows the mannequin to regulate its prompting technique dynamically primarily based on the duty necessities and the person’s enter, resulting in extra adaptive and contextually related interactions.
- Artistic Immediate Era: Meta-prompting explores immediate areas, enabling the mannequin to generate various and revolutionary prompts. It conjures up new heights of thought and expression.
- Process-Particular Immediate Era: Meta-prompting allows the era of prompts tailor-made to particular duties or domains, guaranteeing that the mannequin’s responses align with the person’s intentions and the duty’s necessities.
- Autonomous Immediate Refinement: Meta-prompting permits the mannequin to refine prompts autonomously primarily based on suggestions and expertise. This helps the mannequin repeatedly enhance and refine its prompting technique.
Additionally learn: Prompt Engineering: Definition, Examples, Tips & More
Strategies for Meta-Prompting
- Immediate Era by Instance: Meta-prompting can contain producing prompts primarily based on examples supplied by the person from the duty context. By analyzing these examples, the mannequin identifies related patterns and constructions that inform the era of latest prompts tailor-made to the duty’s particular necessities.
- Immediate Refinement by way of Suggestions: Meta-prompting permits the mannequin to refine prompts iteratively primarily based on suggestions from its personal responses and the person’s enter. This suggestions loop permits the mannequin to be taught from its errors and regulate its prompting technique to enhance the standard of its output over time.
- Immediate Era from Process Descriptions: Meta-prompting can present pure language understanding methods to extract key data from activity descriptions or person queries and use this data to generate prompts tailor-made to the duty at hand. This ensures that the generated prompts are aligned with the person’s intentions and the particular necessities of the duty.
- Immediate Era primarily based on Mannequin State: Meta-prompting generates prompts by taking account of the inner state of the mannequin, together with its information base, reminiscence, and inference capabilities. This occurs by leveraging the mannequin’s present information and reasoning skills. This permits the mannequin to generate contextually related prompts and align with its present state of understanding.
Instance: Producing Prompts for a Process
task_description = "Summarize the important thing factors of a information article."
meta_prompt = f"""
You're an skilled in immediate engineering. Create a immediate for the next activity:
Process: {task_description}
Immediate:
"""
response = mannequin.generate(meta_prompt)
print(response)
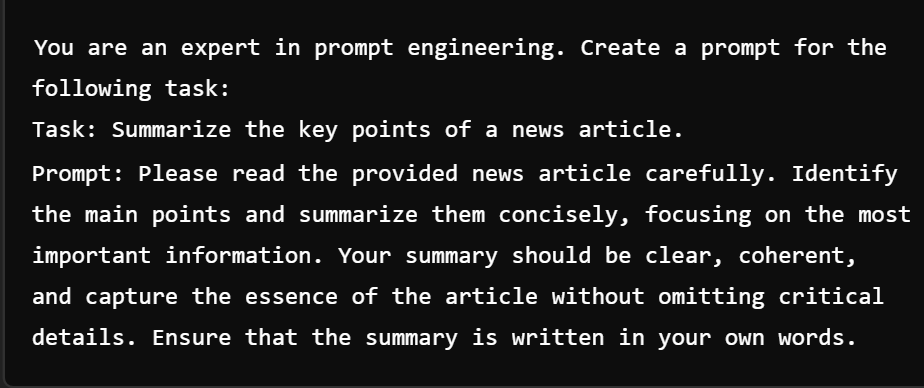
Leveraging Reminiscence and State
Leveraging reminiscence and state inside language fashions allows the mannequin to retain context and data throughout interactions, which helps empower language fashions to exhibit extra human-like behaviors, akin to sustaining conversational context, monitoring dialogue historical past, and adapting responses primarily based on earlier interactions. By including reminiscence and state mechanisms into the prompting course of, builders can create extra coherent, context-aware, and responsive interactions with language fashions.
Functions of Leveraging Reminiscence and State
- Contextual Conversational Brokers: Reminiscence and state mechanisms allow language fashions to behave as context-aware conversational brokers, sustaining context throughout interactions and producing responses which can be coherent with the continued dialogue.
- Personalised Suggestions: On this, language fashions can present customized suggestions tailor-made to the person’s preferences and previous interactions, enhancing the relevance and effectiveness of advice techniques.
- Adaptive Studying Environments: It may possibly improve interactive studying environments by monitoring learners’ progress and adapting the educational content material primarily based on their wants and studying trajectory.
- Dynamic Process Execution: Language fashions can execute complicated duties over a number of interactions whereas coordinating their actions and responses primarily based on the duty’s evolving context.
Strategies for Leveraging Reminiscence and State
- Dialog Historical past Monitoring: Language fashions can keep a reminiscence of earlier messages exchanged throughout a dialog, which permits them to retain context and monitor the dialogue historical past. By referencing this dialog historical past, fashions can generate extra coherent and contextually related responses that construct upon earlier interactions.
- Contextual Reminiscence Integration: Reminiscence mechanisms might be built-in into the prompting course of to offer the mannequin with entry to related contextual data. This helps builders in guiding the mannequin’s responses primarily based on its previous experiences and interactions.
- Stateful Immediate Era: State administration methods permit language fashions to take care of an inner state that evolves all through the interplay. Builders can tailor the prompting technique to the mannequin’s inner context to make sure the generated prompts align with its present information and understanding.
- Dynamic State Replace: Language fashions can replace their inner state dynamically primarily based on new data obtained in the course of the interplay. Right here, the mannequin repeatedly updates its state in response to person inputs and mannequin outputs, adapting its habits in real-time and enhancing its potential to generate contextually related responses.
Instance: Sustaining State in Conversations
from langchain.reminiscence import ConversationBufferMemory
reminiscence = ConversationBufferMemory()
dialog = [
"User: What's the weather like today?",
"AI: The weather is sunny with a high of 25°C.",
"User: Should I take an umbrella?"
]
for message in dialog:
reminiscence.add_message(message)
immediate = f"""
Proceed the dialog primarily based on the next context:
{reminiscence.get_memory()}
AI:
"""
response = mannequin.generate(immediate)
print(response)
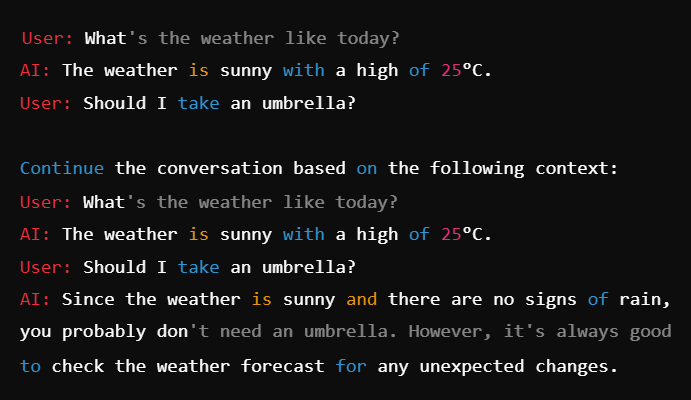
Sensible Examples
Instance 1: Superior Textual content Summarization
Utilizing dynamic and context-aware prompting to summarize complicated paperwork.
#importdocument = """
LangChain is a framework that simplifies the method of constructing purposes utilizing giant language fashions. It offers instruments to create efficient prompts and combine with numerous APIs and knowledge sources. LangChain permits builders to construct purposes which can be extra environment friendly and scalable.
"""
immediate = f"""
Summarize the next doc:
{doc}
Abstract:
"""
response = mannequin.generate(immediate)
print(response)
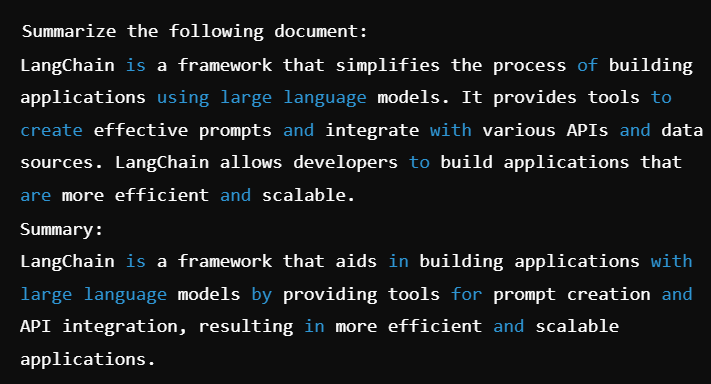
Instance 2: Complicated Query Answering
Combining multi-step reasoning and context-aware prompts for detailed Q&A.
query = "Clarify the speculation of relativity."
immediate = f"""
You're a physicist. Clarify the speculation of relativity in easy phrases.
Query: {query}
Reply:
"""
response = mannequin.generate(immediate)
print(response)
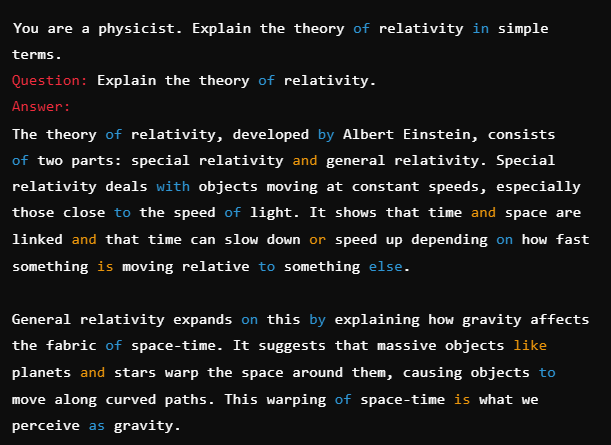
Conclusion
Superior immediate engineering with LangChain helps builders to construct strong, context-aware purposes that leverage the total potential of enormous language fashions. Steady experimentation and refinement of prompts are important for attaining optimum outcomes.
For complete knowledge administration options, discover YData Fabric. For instruments to profile datasets, think about using ydata-profiling. To generate artificial knowledge with preserved statistical properties, try ydata-synthetic.
Key Takeaways
- Superior Immediate Engineering Structuring: Guides mannequin by way of multi-step reasoning with contextual cues.
- Dynamic Prompting: Adjusts prompts primarily based on real-time context and person interactions.
- Context-Conscious Prompts: Evolves prompts to take care of relevance and coherence with dialog context.
- Meta-Prompting: Generates and refines prompts autonomously, leveraging the mannequin’s capabilities.
- Leveraging Reminiscence and State: Maintains context and data throughout interactions for coherent responses.
The media proven on this article should not owned by Analytics Vidhya and is used on the Creator’s discretion.
Frequently Asked Questions
A. LangChain can integrate with APIs and data sources to dynamically adjust prompts based on real-time user input or external data. You can create highly adaptive and context-aware interactions by programmatically constructing prompts incorporating this information.
A. LangChain provides memory management capabilities that allow you to store and retrieve context across multiple interactions, essential for creating conversational agents that remember user preferences and past interactions.
A. Handling ambiguous or unclear queries requires designing prompts that guide the model in seeking clarification or providing context-aware responses. Best practices include:
a. Explicitly Asking for Clarification: Prompt the model to ask follow-up questions.
b. Providing Multiple Interpretations: Design prompts allow the model to present different interpretations.
A. Meta-prompting leverages the model’s own capabilities to generate or refine prompts, enhancing the overall application performance. This can be particularly useful for creating adaptive systems that optimize behavior based on feedback and performance metrics.
A. Integrating LangChain with existing machine learning models and workflows involves using its flexible API to combine outputs from various models and data sources, creating a cohesive system that leverages the strengths of multiple components.