Introduction
All through the ever-evolving cloud computing scene, Microsoft Azure stands out as a sturdy stage that offers a wide range of administrations that disentangle capabilities’ growth, affiliation, and administration. From new corporations to expansive endeavors, engineers leverage Azure to enhance their capabilities with the administration of cloud innovation and manufactured insights. This textual content investigates the utterly totally different capabilities of Microsoft Azure, specializing in how numerous administrations might be coordinated to form environment friendly cloud-based preparations.
Learning Targets
- Get the center administrations marketed by Microsoft Azure and their capabilities in cloud computing.
- Uncover methods to convey and oversee digital machines and administrations utilizing the Purplish blue entrance.
- Select up proficiency in configuring and securing cloud functionality picks inside Azure.
- Grasp implementing and managing Azure AI and machine finding out corporations to bolster utility capabilities.
This textual content was printed as a part of the Data Science Blogathon.
Understanding Microsoft Azure
Microsoft Azure may be an entire cloud computing profit made by Microsoft for establishing, testing, passing on, and directing capabilities and organizations by Microsoft-managed information services. It bolsters utterly totally different programming dialects, apparatuses, and Microsoft-specific strategies and third-party laptop packages.
Azure’s Key Firms: An Illustrated Overview
Azure’s in depth catalog comprises choices like AI and machine learning, databases, and development devices, all underpinned by layers of security and compliance frameworks. To assist understanding, let’s delve into a couple of of those corporations with the help of diagrams and flowcharts that outline how Azure integrates into typical development workflows:
- Azure Compute Firms: Visualize how VMs, Azure Options, and App Firms work collectively contained in the cloud setting.
- Info Administration and Databases: Uncover the construction of Azure SQL Database and Cosmos DB by detailed schematics.
Full Overview of Microsoft Azure Firms and Integration Strategies
Microsoft Azure Overview
Microsoft Azure may be a driving cloud computing stage given by Microsoft, selling an entire suite of administrations for utility to, administration, and enchancment over worldwide information services. This stage underpins a wide array of capabilities, counting Laptop computer program as a Revenue (SaaS), Stage as a Revenue (PaaS), and Infrastructure as a Revenue (IaaS), obliging an assortment of programming dialects, gadgets, and strategies.
Introduction to Azure Firms
Azure offers a great deal of administrations, nevertheless for our instructional practice, we’ll coronary heart on three key parts:
- Azure Blob Storage: Glorious for putting away massive volumes of unstructured information.
- Azure Cognitive Firms: Affords AI-powered textual content material analytics capabilities.
- Azure Doc Intelligence (Kind Recognizer): Permits structured information extraction from paperwork.
Setting Up Your Azure Setting
Sooner than diving into code, assure you’ve got bought:
- An Azure account with entry to these corporations.
- Python put in in your machine.
Preliminary Setup and Imports
First, prepare your Python environment by placing within the obligatory packages and configuring setting variables to attach with Azure corporations.
# Python Setting Setup
import os
from azure.storage.blob import BlobServiceClient
from azure.ai.textanalytics import TextAnalyticsClient
from azure.ai.formrecognizer import DocumentAnalysisClient
from azure.id import DefaultAzureCredential
# Organize setting variables
os.environ["AZURE_STORAGE_CONNECTION_STRING"] = "your_connection_string_here"
os.environ["AZURE_FORM_RECOGNIZER_ENDPOINT"] = "https://your-form-recognizer-resource.cognitiveservices.azure.com/"
os.environ["AZURE_FORM_RECOGNIZER_KEY"] = "your_key_here"
Leveraging Azure Blob Storage
Purplish Blue Blob Functionality is a main service equipped by Microsoft Purplish Blue for putting away expansive sums of unstructured information, akin to content material materials data, footage, recordings, and fairly extra. It is consider to cope with every the overwhelming requests of large-scale capabilities and the info functionality desires of smaller frameworks productively. Underneath, we delve into the way in which to rearrange and take advantage of Azure Blob Storage efficiently.
Setting Up Azure Blob Storage
Step one in leveraging Sky Blue Blob Storage is establishing the fundamental foundation inside your Azure setting. Proper right here’s the way in which to get started:
Create a Storage Account
- Log into your Azure Portal.
- Uncover “Functionality Accounts” and tap on “Make.”
- Fill out the physique by selecting your membership and helpful useful resource group (or create an unused one) and indicating the attention-grabbing title in your functionality account.
- Select the realm closest to your shopper base for finest execution.
- Select an execution diploma (Commonplace or Premium) relying in your funds and execution necessities.
- Analysis and create your storage account.
Arrange Info into Containers
- As quickly as your functionality account is about up, it’s best to create holders inside it, which act like catalogs to rearrange your data.
- Go to your functionality account dashboard, uncover the “Blob revenue” half, and press on “Holders”.
- Faucet on “+ Container” to make a recent one. Specify a status in your container and set the entry diploma (personal, blob, or container) counting on the way in which you need to deal with entry to the blobs saved inside.
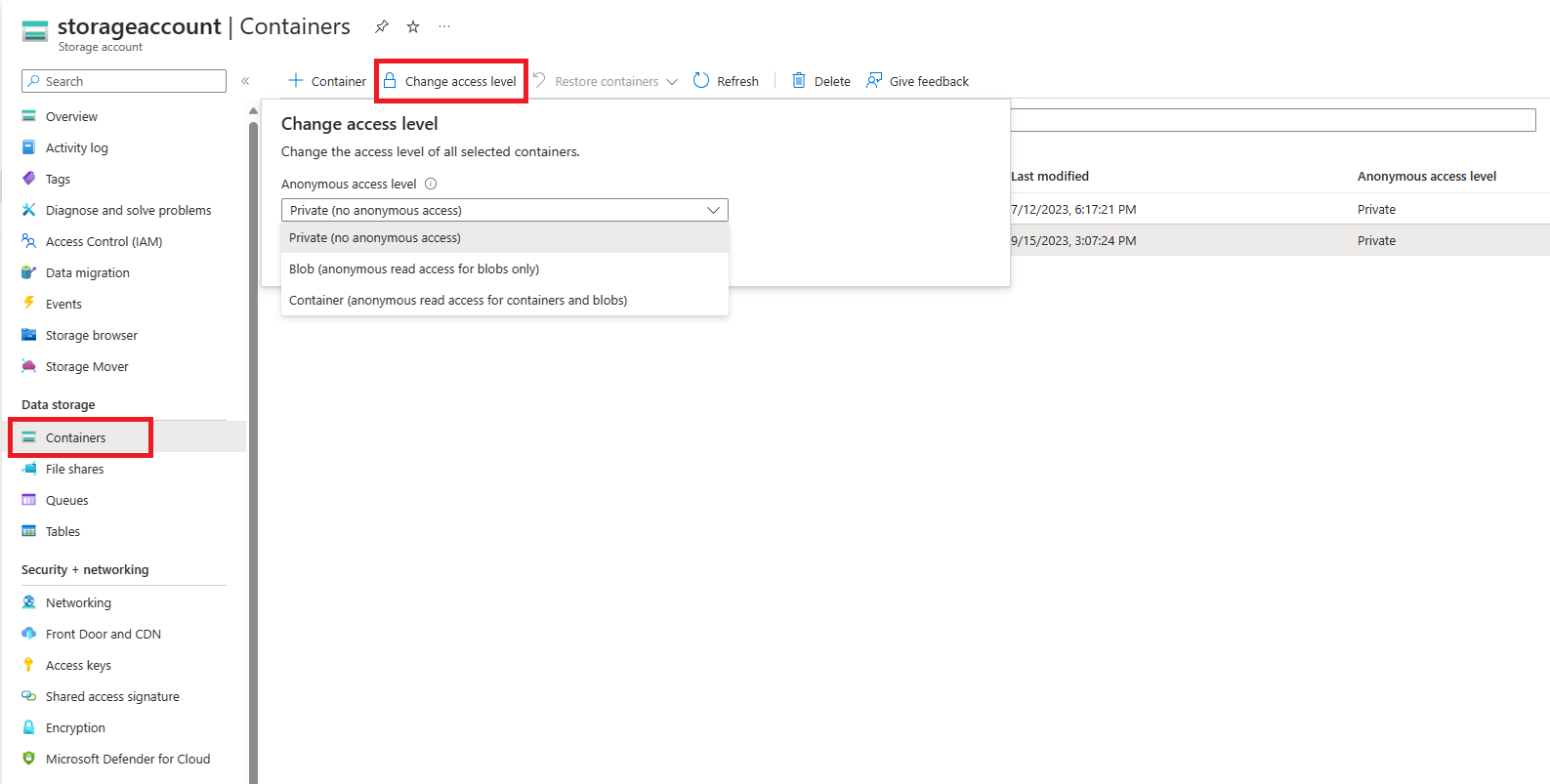
Wise Implementation
Collectively together with your Azure Blob Storage ready, proper right here’s the way in which to implement it in a wise state of affairs using Python. This occasion demonstrates the way in which so as to add, guidelines, and acquire blobs.
# Importing Info to Blob Storage
def upload_file_to_blob(file_path, container_name, blob_name):
connection_string = os.getenv('AZURE_STORAGE_CONNECTION_STRING')
blob_service_client = BlobServiceClient.from_connection_string(connection_string)
blob_client = blob_service_client.get_blob_client(container=container_name, blob=blob_name)
with open(file_path, "rb") as information:
blob_client.upload_blob(information)
print(f"File {file_path} uploaded to {blob_name} in container {container_name}")
# Occasion Utilization
upload_file_to_blob("occasion.txt", "example-container", "example-blob")
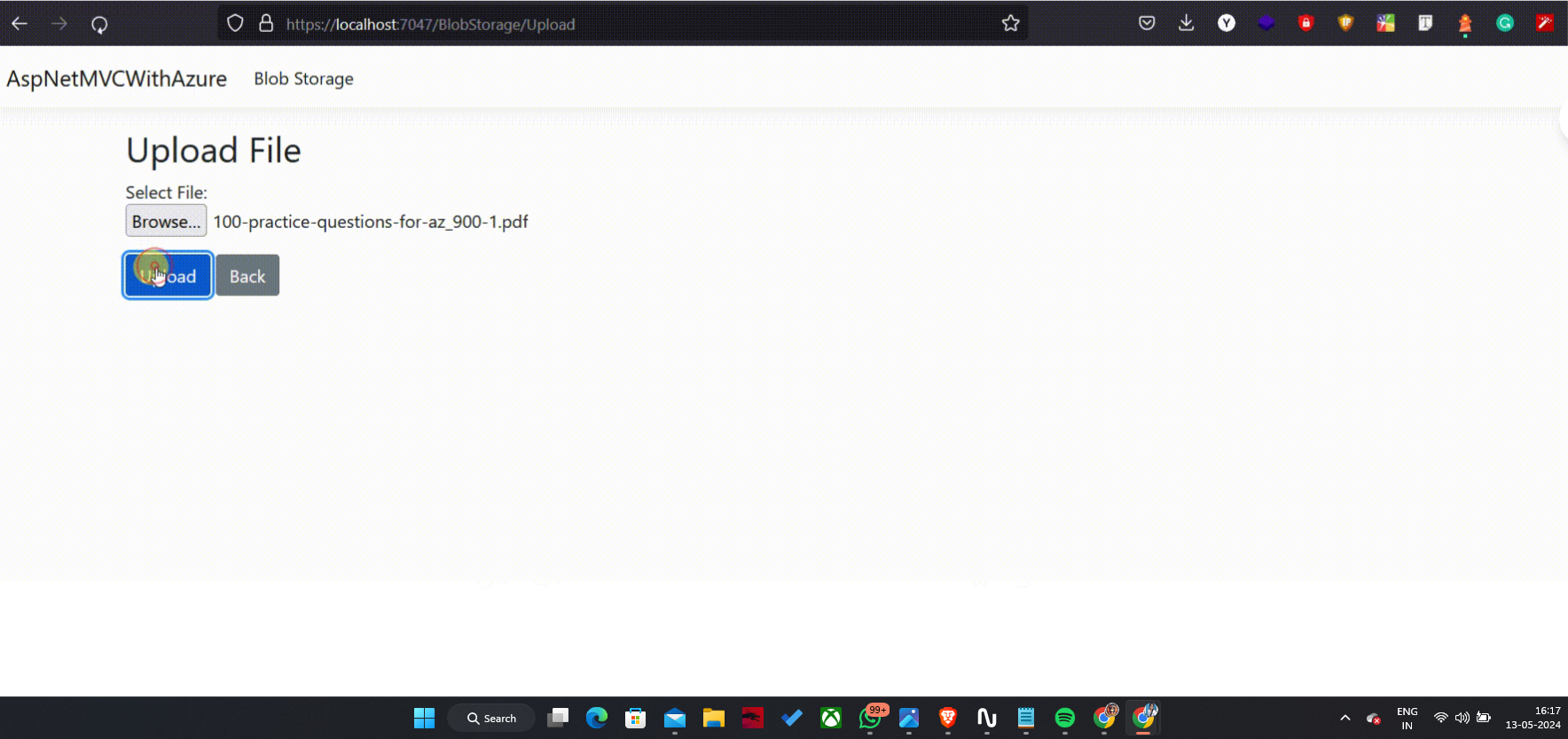
These operations symbolize merely the ground of what Azure Blob Storage can do. The service moreover helps superior choices akin to snapshots, blob versioning, lifecycle administration insurance coverage insurance policies, and fine-grained entry controls, making it a brilliant different for sturdy data management desires.
Analyzing Textual content material Info with Azure Cognitive Firms
Azure Cognitive Services, considerably Textual content material Analytics, offers extremely efficient devices for textual content material analysis.
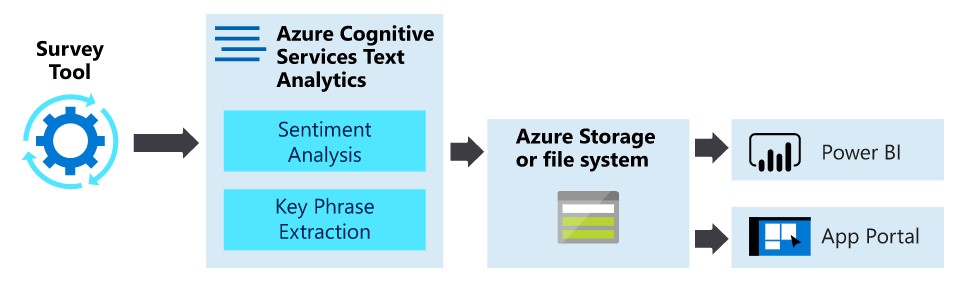
Key Choices
- Estimation Examination: This highlights the tone and feeling handed on in a physique of content material materials. It classifies constructive, unfavourable, and impartial opinions, giving an estimation score for each doc or content material materials bit. This shall be considerably useful for gauging shopper sentiment in surveys or social media.
- Key Particular Extraction: Key categorical extraction acknowledges in all probability probably the most focuses and topics in content material materials. By pulling out vital expressions, this instrument helps to shortly get the quintessence of huge volumes of content material materials with out the requirement for handbook labeling or broad perusing.
- Substance Acknowledgment: This usefulness acknowledges and categorizes substances inside content material materials into predefined lessons akin to specific individual names, areas, dates, and so forth. Substance acknowledgment is efficacious for quickly extricating very important information from content material materials, akin to sorting data articles by geological significance or determining crucial figures in substance.
Integration and Utilization
Integrating Azure Textual content material Analytics into your capabilities comprises establishing the revenue on the Purplish blue stage and utilizing the given SDKs to hitch content material materials examination highlights into your codebase. Proper right here’s the way in which you’ll get started:
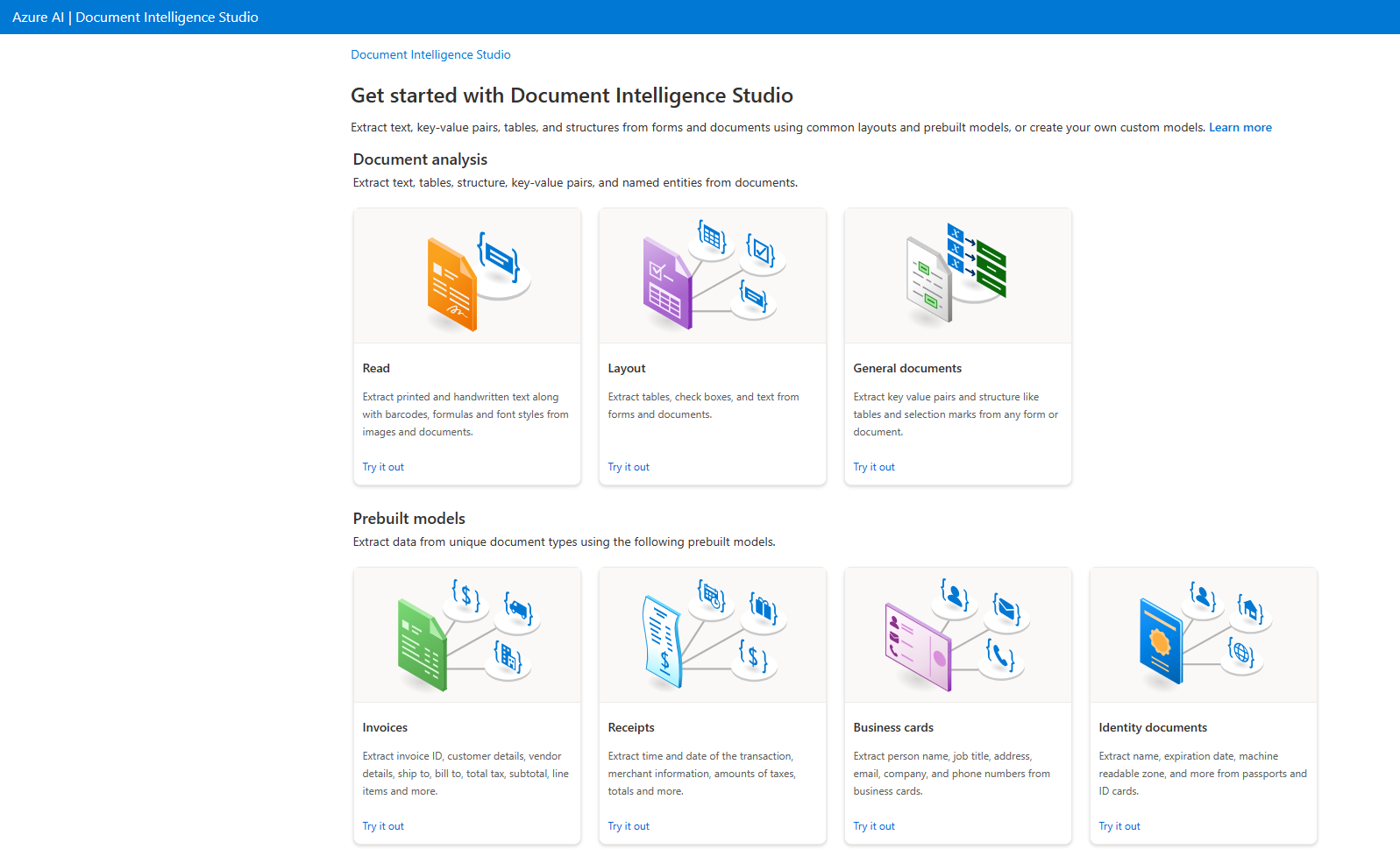
Create a Textual content material Analytics Helpful useful resource
- Log into your Azure portal.
- Create a model new Textual content material Analytics helpful useful resource, selecting the acceptable subscription and helpful useful resource group. After configuration, Azure will current an endpoint and a key, which can be vital for accessing the service.
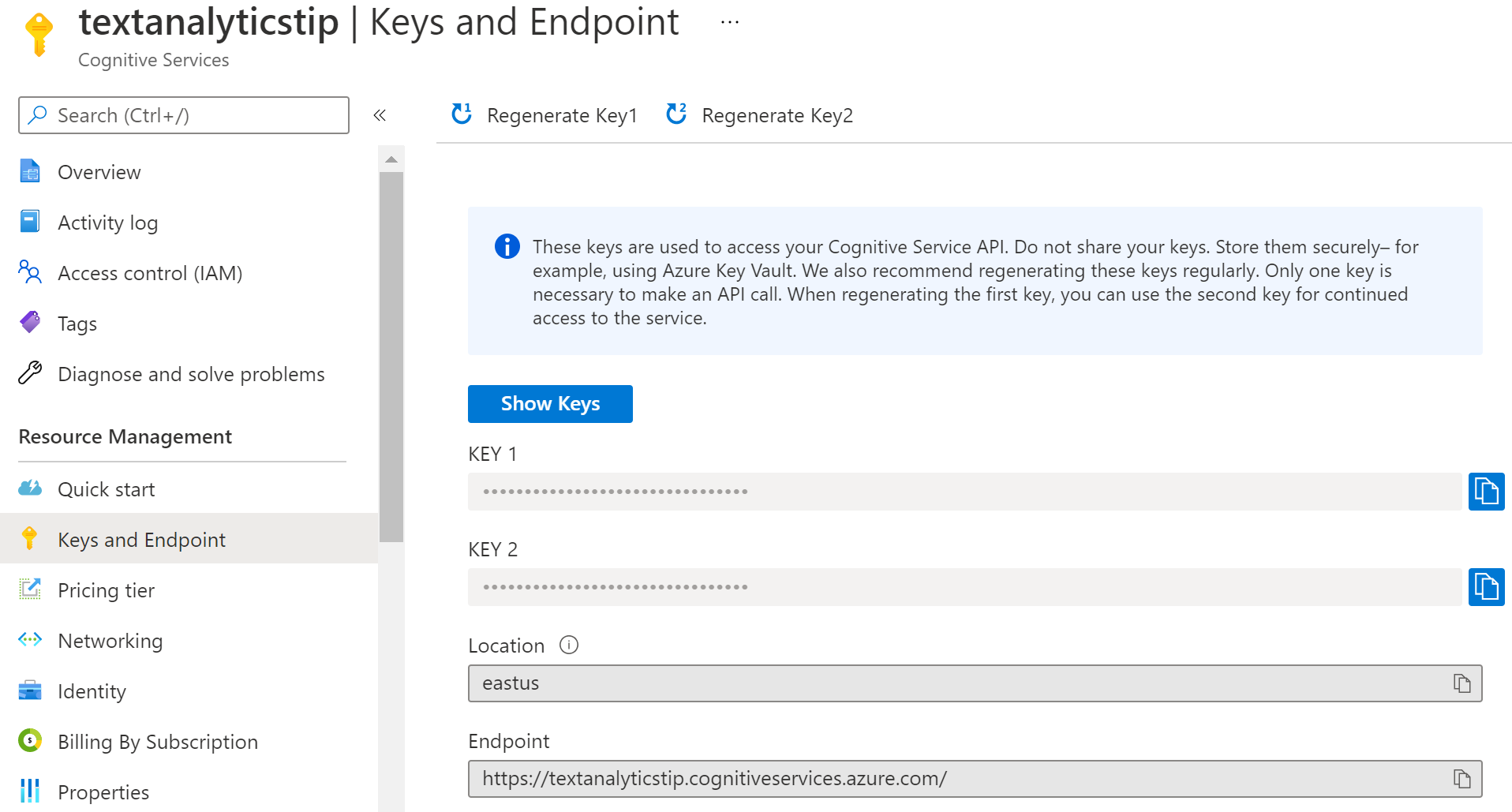
# Analyzing Sentiment
def analyze_sentiment(textual content material):
endpoint = os.getenv("AZURE_TEXT_ANALYTICS_ENDPOINT")
key = os.getenv("AZURE_TEXT_ANALYTICS_KEY")
text_analytics_client = TextAnalyticsClient(endpoint=endpoint, credential=AzureKeyCredential(key))
paperwork = [text]
response = text_analytics_client.analyze_sentiment(paperwork=paperwork)
for doc in response:
print(f"Doc Sentiment: {doc.sentiment}")
print(f"Scores: Optimistic={doc.confidence_scores.constructive:.2f}, Neutral={doc.confidence_scores.neutral:.2f}, Unfavorable={doc.confidence_scores.unfavourable:.2f}")
# Occasion Utilization
analyze_sentiment("Azure AI Textual content material Analytics affords extremely efficient pure language processing over raw textual content material.")
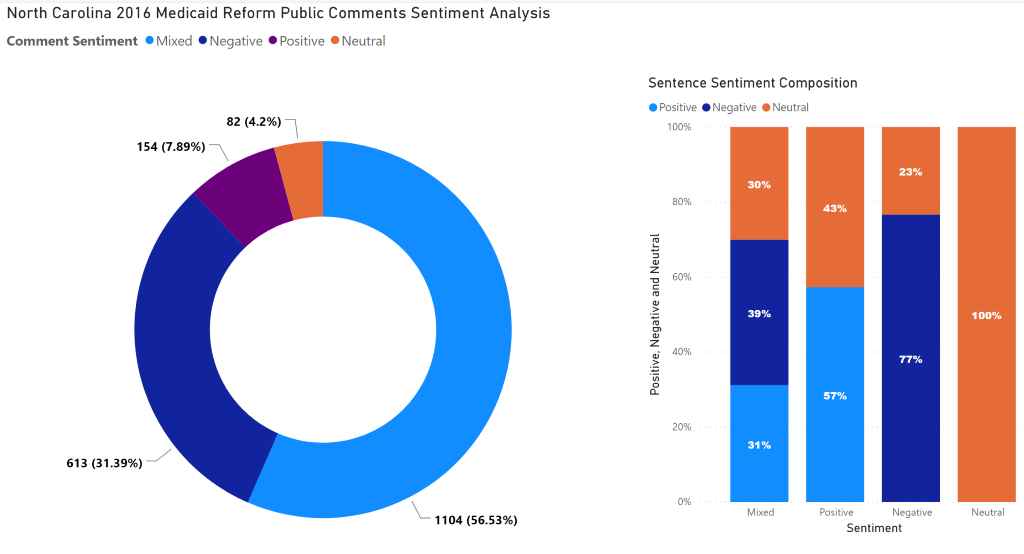
By coordinating these capabilities, you’ll be able to improve your capabilities with profound bits of knowledge determined from literary information, empowering additional educated decision-making and giving wealthier, additional intuitive shopper involvement. Whether or not or not for estimation monitoring, content material materials summarization, or information extraction, Sky Blue Cognitive Firms’ Content material materials Analytics offers an entire affiliation to fulfill the utterly totally different desires of current capabilities.
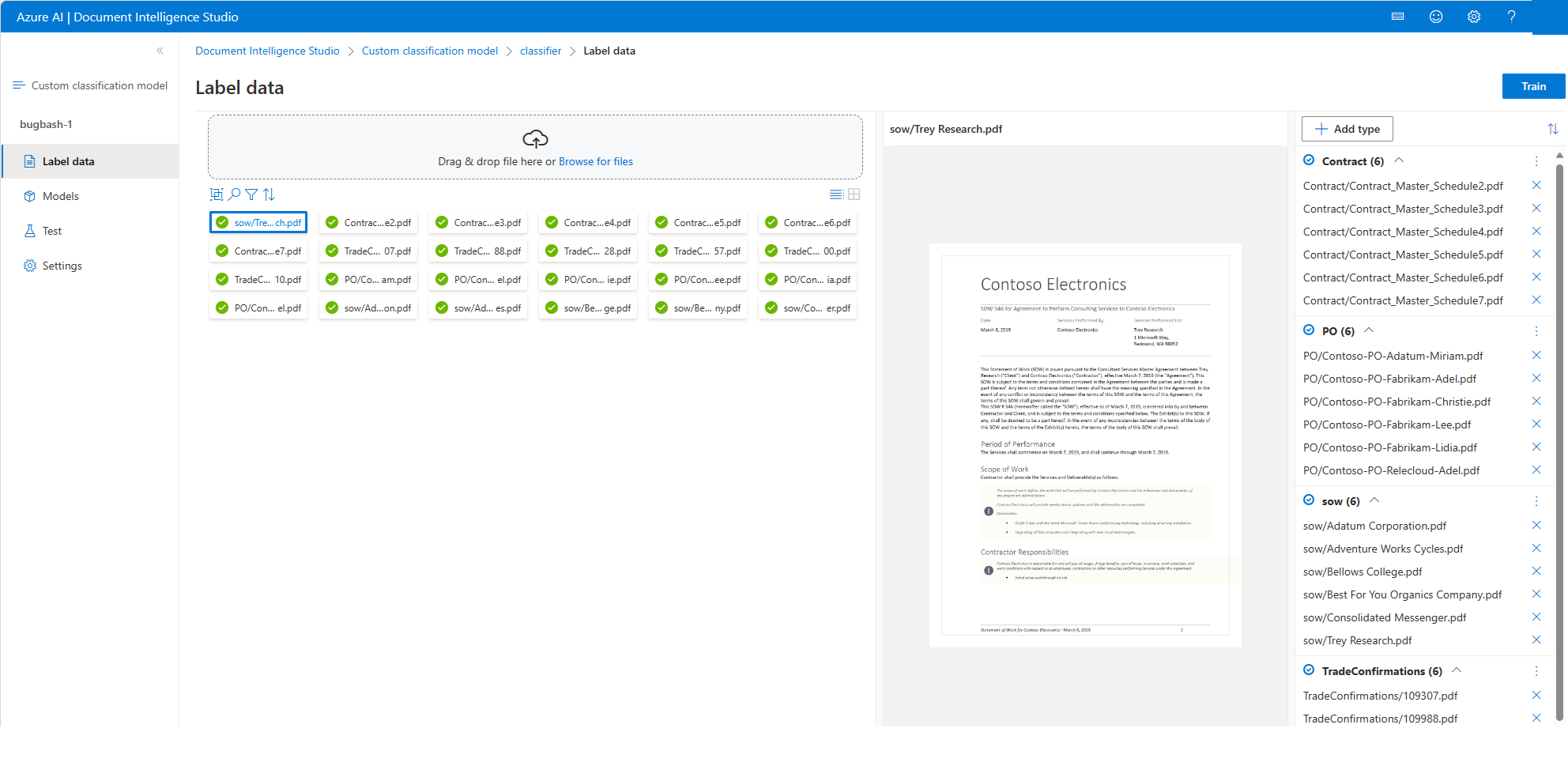
- Report Administration: The interface permits consumers to efficiently oversee and prepare archives by dragging and dropping them into the studio or utilizing the browse totally different to modify data.
- Personalized Classification Show: Consumers can label and categorize numerous sorts of research akin to contracts, buy orders, and additional to rearrange personalized classification fashions.
- Visualization of Doc Info: The platform reveals detailed views of chosen paperwork, such as a result of the “Contoso Electronics” doc, showcasing the studio’s capabilities for in-depth analysis and training.
- Interactive UI Choices: The UI helps diverse doc varieties, with devices for together with, labeling, and managing doc information efficiently, enhancing individual interaction and effectivity in information coping with.
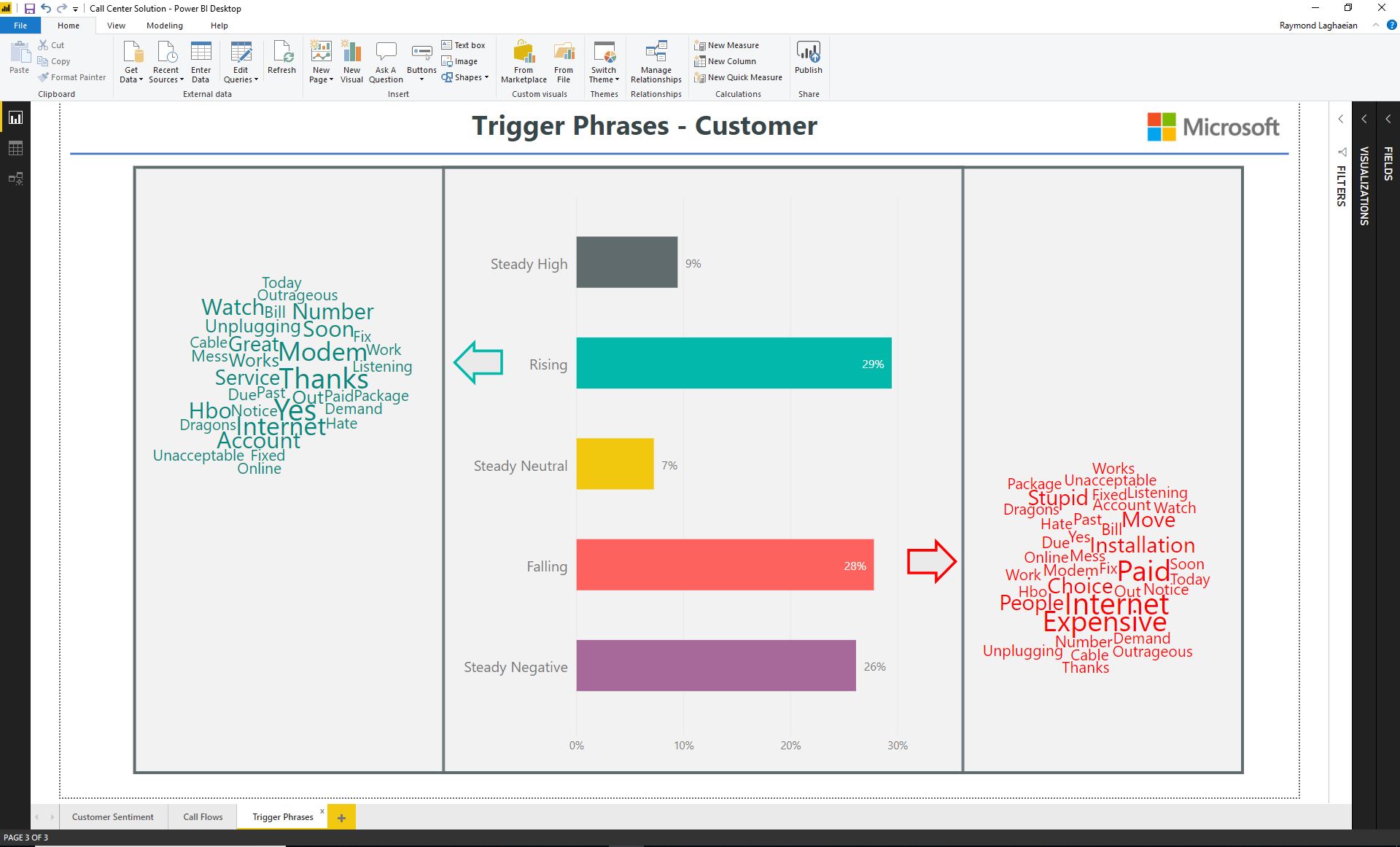
Utilizing Azure Doc Intelligence (Kind Recognizer)
Azure Kind Recognizer might probably be an environment friendly instrument inside Microsoft Azure’s suite of AI administrations. It makes use of machine finding out procedures to extract organized information from doc groups. This revenue is designed to remodel unstructured paperwork into usable, organized information, empowering computerization and proficiency in diverse commerce sorts.
Key Capabilities
Azure Kind Recognizer comprises two principal sorts of model capabilities:
- Prebuilt Fashions: These can be found and expert to hold out specific duties, akin to extracting information from invoices, receipts, enterprise enjoying playing cards, and kinds, with out additional teaching. They’re good for frequent doc processing duties, allowing for quick utility integration and deployment.
- Personalized Fashions: Kind Recognizer permits clients to teach personalized fashions for additional specific desires or paperwork with distinctive codecs. These fashions might be tailored to acknowledge information varieties from paperwork specific to a specific enterprise or enterprise, offering extreme customization and flexibility.
The smart utility of Azure Kind Recognizer might be illustrated by a Python carry out that automates the extraction of knowledge from paperwork. Underneath is an occasion demonstrating the way in which to make use of this service to analysis paperwork akin to invoices:
# Doc Analysis with Kind Recognizer
def analyze_document(file_path):
endpoint = os.getenv('AZURE_FORM_RECOGNIZER_ENDPOINT')
key = os.getenv('AZURE_FORM_RECOGNIZER_KEY')
form_recognizer_client = DocumentAnalysisClient(endpoint=endpoint, credential=AzureKeyCredential(key))
with open(file_path, "rb") as f:
poller = form_recognizer_client.begin_analyze_document("prebuilt-document", doc=f)
end result = poller.end result()
for idx, doc in enumerate(end result.paperwork):
print(f"Doc #{idx+1}:")
for title, self-discipline in doc.fields.objects():
print(f"{title}: {self-discipline.value} (confidence: {self-discipline.confidence})")
# Occasion Utilization
analyze_document("invoice.pdf")
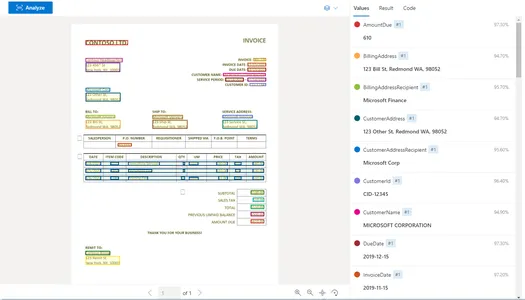
This work illustrates how Sky Blue Kind Recognizer can streamline the tactic of extricating key information from research, which can then be coordinated into utterly totally different workflows akin to accounts payable, shopper onboarding, or each different document-intensive cope with. By robotizing these assignments, corporations can diminish handbook errors, increment proficiency, and coronary heart property on additional crucial exercises.
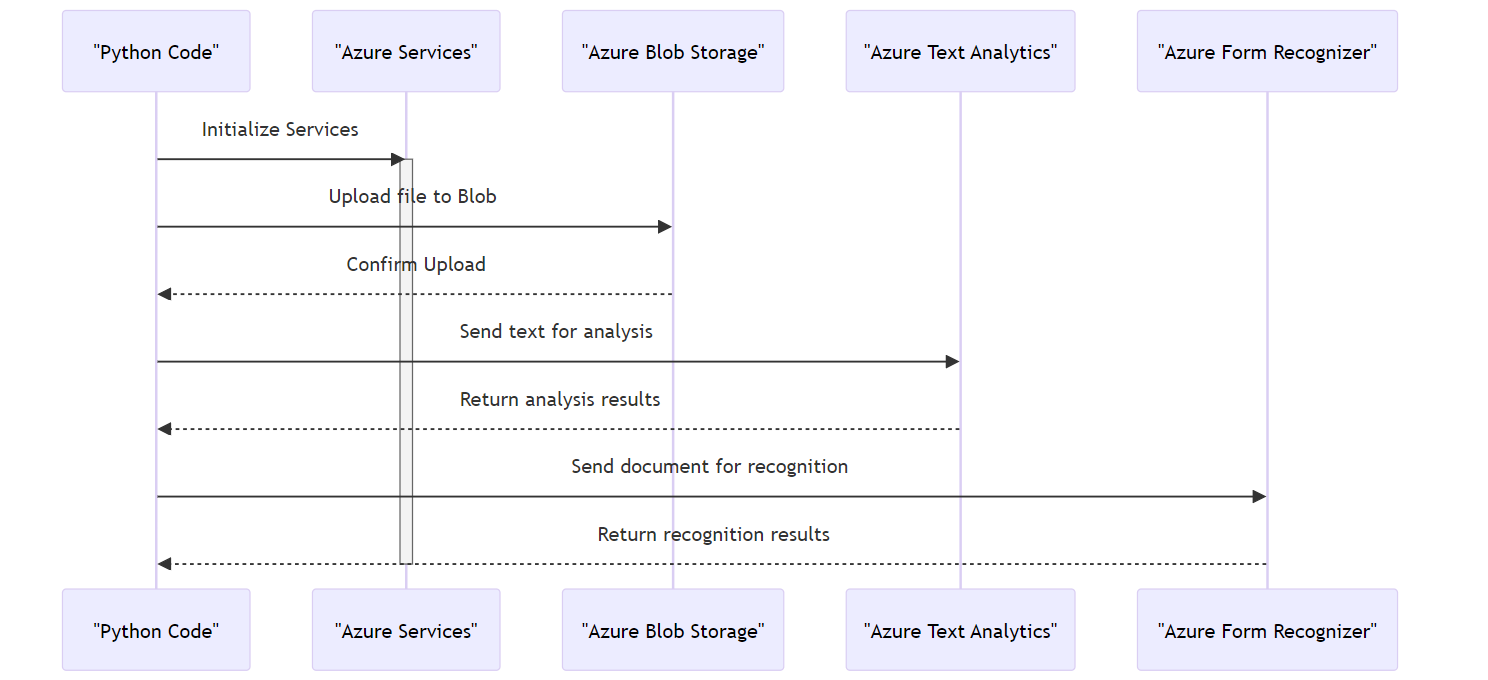
Integration All through Firms
Combining Azure corporations enhances efficiency:
- Retailer paperwork in Blob Storage and course of them with Kind Recognizer.
- Analyze content material materials information extricated from research utilizing Content material materials Analytics.
By changing into a member of Azure With Blob Functionality, Cognitive Administrations, and Archive Insights, school college students can purchase an entire affiliation for information administration and investigation.
Coordination of varied Azure administrations can improve capabilities’ capabilities by leveraging the one-of-a-kind qualities of each revenue. This synergistic technique streamlines workflows and improves information coping with and expository accuracy. Proper right here’s a nitty gritty see at how combining Azure Blob Functionality, Cognitive Administrations, and Report Insights might make a succesful setting for full information administration and examination:
- Report Functionality and Coping with: Azure Blob Functionality is a perfect retailer for putting away infinite sums of unstructured information and counting archives in a number of designs like PDFs, Phrase data, and pictures. As quickly as put away, these archives might be always able to take advantage of Azure Type Recognizer, which extricates content material materials and information from the archives. Physique Recognizer applies machine finding out fashions to get the doc development and extricate key-value models and tables, turning filtered research into noteworthy, organized information.
- Progressed Content material materials Examination: Azure Cognitive Firms’ Content material materials Analytics can encourage substance analysis after extricating content material materials from data. This service affords superior pure language processing over the raw textual content material extracted by Kind Recognizer. It might probably decide the concept of the content material materials, acknowledge key expressions, acknowledge named substances akin to dates, of us, and places, and definitely set up the dialect of the content material materials. This step is vital for capabilities requiring opinion examination, shopper criticism investigation, or each different type of content material materials translation that helps in decision-making.
The mix of these administrations not solely robotizes the info dealing with preparation however moreover upgrades information top quality by progressed analytics. This combined technique permits school college students and builders to:
- Diminish Information Exertion: Robotizing the extraction and investigation of knowledge from archives decreases the handbook information half and audit requirement, minimizing blunders and rising proficiency.
- Enhance Willpower Making: With additional right and nicely timed information extraction and analysis, school college students and builders might make better-informed picks based totally on data-driven insights.
- Scale Choices: As desires develop, Azure’s scalability permits the coping with of an rising amount of data with out sacrificing effectivity, making it acceptable for tutorial initiatives, evaluation, and enterprise capabilities alike.
Moreover study: Azure Machine Learning: A Step-by-Step Guide
Benefits of Using Azure
The mix of Azure corporations affords a number of benefits:
- Versatility: Azure’s foundation permits capabilities to scale on-demand, pleasing changes in utilization with out forthright speculations.
- Security: Constructed-in security controls and progressed risk analytics make sure that capabilities and information are protected in direction of potential dangers.
- Innovation: With entry to Azure’s AI and machine finding out corporations, corporations can always innovate and improve their corporations.
Precise-World Success: Azure in Movement
To solidify the smart implications of adopting Azure, take into consideration the tales of corporations which have transitioned to Azure:
- E-commerce Monster Leverages Azure for Adaptability: A driving on-line retailer utilized Azure’s compute capabilities to cope with variable lots of amid prime buying seasons, outlining the platform’s versatility and unwavering top quality.
- Healthcare Supplier Improves Understanding Administrations with Azure AI: A healthcare supplier executed Purplish blue AI apparatuses to streamline quiet information coping with and progress symptomatic exactness, exhibiting Azure’s impression on revenue conveyance and operational effectiveness.
Comparative Analysis: Azure vs. Rivals
Whereas Azure offers a sturdy set of administrations, how does it stack up in direction of rivals like AWS and Google Cloud?
- Revenue Breadth and Profundity: Consider the number of administrations and the profundity of highlights over Azure, AWS, and Google Cloud.
- Estimating Adaptability: Analyze the estimating fashions of each stage to find out which supplies in all probability probably the most fantastic cost-efficiency for distinctive take advantage of cases.
- Half-breed Cloud Capabilities: Assess each provider’s preparations for coordination with on-premises environments—a key thought for fairly a couple of corporations.
- Innovation and Ecosystem: Concentrate on the innovation monitor doc and the ability of the developer ecosystem surrounding each platform.
Conclusion
Microsoft Azure offers an brisk and adaptable setting that will cater to the quite a few desires of present-day capabilities. By leveraging Azure’s full suite of administrations, corporations can assemble additional clever, responsive, and versatile capabilities. Whether or not or not it’s by upgrading information administration capabilities or changing into a member of AI-driven bits of knowledge, Azure offers the devices vital for corporations to flourish contained in the computerized interval.
By understanding and utilizing these administrations effectively, engineers can guarantee they’re on the forefront of mechanical developments, making optimum use of cloud computing property to drive commerce victory.
Key Takeaways
- Full Cloud Preparations: Microsoft Azure offers diverse low cost administrations for numerous capabilities, along with AI and machine finding out, information administration, and cloud computing. This flexibility makes it a perfect different for corporations using cloud innovation over utterly totally different operational zones.
- Personalized fitted for Specialised Consultants: The article considerably addresses designers and IT consultants, giving specialised experiences, code cases, and integration procedures which could be straightforwardly relevant to their day-to-day work.
- Seen Help Enhance Understanding: By way of using charts, flowcharts, and screenshots, the article clarifies superior concepts and fashions, making it a lot much less demanding for purchasers to know the way Azure administrations might be built-in into their ventures.
- Precise-World Functions: Along with case analysis demonstrates Azure’s smart benefits and real-world efficacy. These examples current how diverse industries effectively implement Azure to boost scalability, effectivity, and innovation.
- Aggressive Analysis: The comparative analysis with AWS and Google Cloud offers a balanced view, serving to readers understand Azure’s distinctive advantages and points as compared with totally different principal cloud platforms. This analysis is important for educated decision-making in cloud service alternative.
The media confirmed on this text shouldn’t owned by Analytics Vidhya and is used on the Creator’s discretion.
Incessantly Requested Questions
A. Microsoft Azure offers an entire suite of cloud administrations, along with nevertheless not restricted to digital computing (Azure Digital Machines), AI and machine finding out (Purplish blue AI), database administration (Sky blue SQL Database, Universe DB), and fairly a couple of others. These administrations cater to quite a few desires akin to analytics, functionality, organizing, and growth, supporting diverse programming dialects and strategies.
A. Azure stands out with its sturdy integration with Microsoft merchandise and administrations, making it notably alluring for organizations that depend on Microsoft packages. Compared with AWS and Google Cloud, Azure ceaselessly offers greater preparations for crossover cloud circumstances and enterprise-level administrations. Pricing fashions might differ, with Azure providing aggressive decisions, considerably in eventualities involving totally different Microsoft software program program.
A. Utterly. Azure should not be sincere for giant ventures; it offers versatile preparations that will develop collectively alongside together with your commerce. New corporations and small corporations revenue from Azure’s pay-as-you-go estimating current, which permits them to pay as a result of it have been for what they take advantage of, minimizing forthright costs. Furthermore, Azure offers gadgets and administrations that will shortly scale as commerce develops.
A. Azure offers utterly totally different benefits for utility enchancment, counting fixed integration with enchancment gadgets, an infinite cluster of programming dialects and strategies bolster, and vigorous adaptability picks. Engineers can use Azure DevOps for ceaseless integration and nonstop conveyance (CI/CD) administrations and Purplish blue Kubernetes Revenue (AKS) to oversee containerized capabilities and improve effectivity and operational effectivity.
A. Microsoft Azure offers considered one of many foremost secure cloud computing circumstances accessible as of late. It has fairly a couple of compliance certifications for locales over the globe. Purplish blue offers built-in security highlights counting organized security, risk assurance, information security, and persona administration preparations. Sky blue consumers can moreover reap the advantages of Azure’s Security Center to get proposals and make strides of their security pose.